::ShowWindow 显示的数据转成cv::Mat
时间: 2023-07-02 08:05:21 浏览: 104
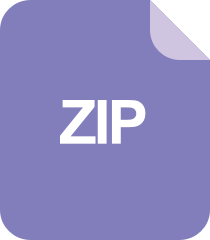
Vue2 全家桶 + Vant 搭建大型单页面商城项目 新蜂商城前床分离版本-前端Vue 项目源码.zip
如果你想将 `ShowWindow` 函数显示的图像数据转换为 `cv::Mat` 类型,可以按照以下步骤操作:
1. 首先,你需要知道 `ShowWindow` 函数显示的图像格式是什么。假设它显示的是 RGB 格式的图像,每个像素用三个字节表示(即每个像素有红、绿、蓝三个通道)。那么你需要知道图像的宽度和高度,以及每行像素的字节数。
2. 接下来,你需要创建一个 `cv::Mat` 对象,以便存储图像数据。你可以使用如下代码创建一个空白的 `cv::Mat` 对象:
```
cv::Mat img(height, width, CV_8UC3);
```
其中,`height` 和 `width` 分别是图像的高度和宽度,`CV_8UC3` 表示每个像素用三个 8 位无符号整数表示。
3. 然后,你需要将 `ShowWindow` 函数返回的图像数据复制到 `cv::Mat` 对象中。你可以使用 `memcpy` 函数将数据复制到 `cv::Mat` 对象的数据指针中,如下所示:
```
memcpy(img.data, imageData, height * width * 3);
```
其中,`imageData` 是 `ShowWindow` 函数返回的图像数据指针,`height * width * 3` 是图像数据的总字节数。
完成上述步骤后,`cv::Mat` 对象中就存储了 `ShowWindow` 函数显示的图像数据。
阅读全文
相关推荐
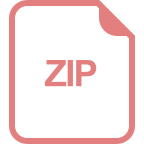
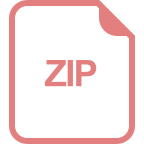
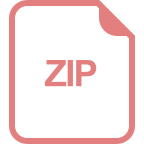
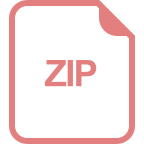
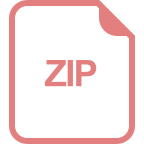
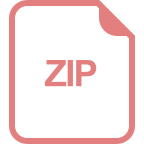
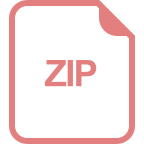
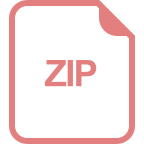
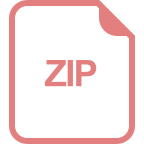
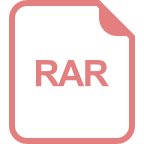
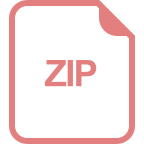
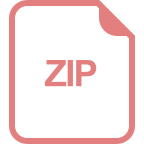
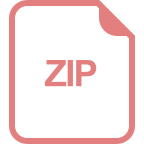
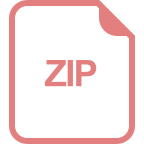
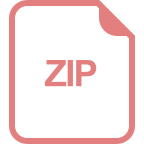