如何代码读取一个txt文件的内容
时间: 2024-06-22 22:02:30 浏览: 165
在编程中,读取文本文件(如.txt格式)的内容通常涉及文件操作,不同编程语言的语法可能会有所不同。以下是使用几种常见编程语言来读取txt文件内容的基本示例:
1. **Python** (使用内置`open()`函数):
```python
with open('filename.txt', 'r') as file:
content = file.read()
print(content)
```
2. **Java** (使用`BufferedReader`):
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
BufferedReader reader = null;
try {
reader = new BufferedReader(new FileReader("filename.txt"));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (reader != null) reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
3. **C#** (使用`StreamReader`):
```csharp
using System.IO;
string[] lines = File.ReadAllLines("filename.txt");
foreach (string line in lines) {
Console.WriteLine(line);
}
```
4. **JavaScript** (Node.js环境,使用`fs`模块):
```javascript
const fs = require('fs');
fs.readFile('filename.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
```
5. **C++** (使用`fstream`库):
```cpp
#include <fstream>
#include <string>
std::ifstream file("filename.txt");
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
```
阅读全文
相关推荐


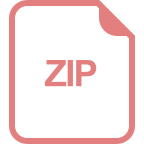







