晴从键盘输入一个字符串(字符串中不包含空格),当输入回车时认为输入结束。 统计输入字符串中的小写英文字母、大写英文字母、数字字符、其他字符的个数。
时间: 2024-11-27 18:10:34 浏览: 24
为了统计输入字符串中的小写英文字母、大写英文字母、数字字符和其他字符的数量,你可以编写如下的Python程序:
```python
def count_chars(input_string):
lowercase = 0
uppercase = 0
digits = 0
others = 0
for char in input_string:
if char.islower():
lowercase += 1
elif char.isupper():
uppercase += 1
elif char.isdigit():
digits += 1
else:
others += 1
return lowercase, uppercase, digits, others
input_str = input("请输入一个字符串(不含空格),按回车键结束输入:")
result = count_chars(input_str)
print(f"小写字母有 {result[0]} 个,大写字母有 {result[1]} 个,数字字符有 {result[2]} 个,其他字符有 {result[3]} 个.")
相关问题
c语言中晴从键盘输入一个字符串(字符串中不包含空格),当输入回车时认为输入结束。 统计输入字符串中的小写英文字母、大写英文字母、数字字符、其他字符的个数。
在C语言中,你可以通过` getchar()`函数配合` while`循环来实现这个功能。这里是一个简单的示例程序:
```c
#include <stdio.h>
#include <ctype.h> // 包含处理字符类型的库
int main() {
char input[100]; // 定义一个足够大的数组来存储输入的字符串
int lowercase = 0; // 计数小写字母
int uppercase = 0; // 计数大写字母
int digit = 0; // 计数数字字符
int other = 0; // 计数其他字符
printf("请输入一个字符串(不含空格,按回车结束):");
while ((input[strlen(input)] != '\n') && (input[strlen(input) - 1] != '\0')) { // 当遇到换行符或字符串结尾才停止
input[strlen(input)] = getchar(); // 读取下一个字符
if (islower(input[strlen(input)])) { // 判断是否为小写字母
lowercase++;
} else if (isupper(input[strlen(input)])) { // 判断是否为大写字母
uppercase++;
} else if (isdigit(input[strlen(input)])) { // 判断是否为数字
digit++;
} else { // 其他字符(包括空格)
other++;
}
}
printf("\n统计结果如下:\n");
printf("小写字母: %d\n", lowercase);
printf("大写字母: %d\n", uppercase);
printf("数字字符: %d\n", digit);
printf("其他字符(包括空格): %d\n", other);
return 0;
}
```
请从键盘输入一个字符串(字符串中不包含空格),当输入回车时认为输入结束。 统计输入字符串中的小写英文字母、大写英文字母、数字字符、其他字符的个数。
当你需要从用户通过键盘输入一个字符串并进行分析时,可以按照以下步骤进行:
1. 首先创建四个计数器变量,分别用于记录小写字母、大写字母、数字字符和其它字符的数量。将它们初始化为0。
```python
count_lowercase = 0
count_uppercase = 0
count_digits = 0
count_other = 0
```
2. 使用一个`while`循环来持续接收用户的输入,直到用户按下回车键。每次循环中,检查输入的字符:
- 对于小写字母,你可以使用`islower()`函数检查,并增加计数器。
- 对于大写字母,使用`isupper()`函数。
- 对于数字,使用`isdigit()`函数。
- 其他字符(包括标点符号、空格等),可以用`isalnum()`判断是否为字母或数字,否则加上`count_other`。
```python
char = input() # 获取一个字符
while char != "":
if char.islower():
count_lowercase += 1
elif char.isupper():
count_uppercase += 1
elif char.isdigit():
count_digits += 1
else:
count_other += 1
char = input()
```
3. 循环结束后,你得到了每个字符类型的计数值。
完整代码示例(Python):
```python
def analyze_input():
count_lowercase = 0
count_uppercase = 0
count_digits = 0
count_other = 0
while True:
char = input()
if char == "":
break
if char.islower():
count_lowercase += 1
elif char.isupper():
count_uppercase += 1
elif char.isdigit():
count_digits += 1
else:
count_other += 1
print(f"小写字母: {count_lowercase}")
print(f"大写字母: {count_uppercase}")
print(f"数字字符: {count_digits}")
print(f"其他字符: {count_other}")
analyze_input()
```
阅读全文
相关推荐
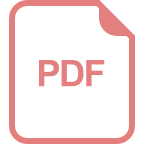
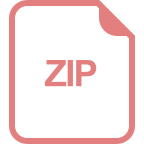
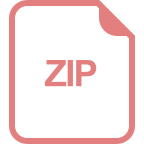













