modbus_tcp_accept如何强行退出执行
时间: 2024-08-08 11:01:42 浏览: 167
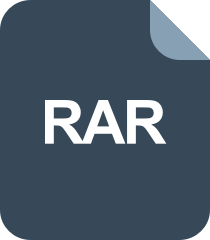
modbus_tcp_monitor_breaker.rar_modbus_tcp
`modbus_tcp_accept()`函数是Modbus库的一部分,用于接收基于TCP/IP协议的Modbus连接请求。如果需要从程序中强制退出此函数的执行过程,实际上并不直接涉及到`modbus_tcp_accept()`自身的一个退出机制,因为这个函数是在等待一个新的客户端连接的到来。
然而,如果你想要在特定条件下中断该等待流程(例如在接收到信号、异常或者其他事件时),你可以通过以下步骤:
1. **设置线程监控**:
使用多线程编程模型,在`modbus_tcp_accept()`运行的线程外部设置一个监控进程或回调函数。一旦满足某种条件(如接收到特定信号、定时器到期等),就调用这个进程或回调函数。
2. **利用系统信号**:
可以捕获并处理系统的中断信号(例如SIGINT 或 SIGTERM)。当接收到此类信号时,可以立即终止`modbus_tcp_accept()`函数的执行,并清理相关的资源。
3. **超时控制**:
在调用`modbus_tcp_accept()`之前,预先设定一个超时时间。可以通过循环调用其他函数(如`select()`, `poll()` 或 `epoll()`)来定期检查是否有新的连接请求到来,同时确保不会无限期地阻塞。
4. **动态资源管理**:
如果你正在使用资源池或其他类型的动态分配策略,可以设计一种机制使得在满足某些条件(比如系统负载过高、可用资源耗尽等)时主动释放资源,并结束`modbus_tcp_accept()`的等待状态。
5. **使用条件变量和互斥锁**:
将`modbus_tcp_accept()`的执行置于条件变量的保护之下。通过条件变量的信号传递机制,可以在满足特定条件时唤醒等待中的线程或停止其运行。
下面是一个简化的示例,展示了如何在多线程环境中使用信号来中断`modbus_tcp_accept()`等待操作:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <signal.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
// Assume we have a Modbus server setup here
int main() {
// Initialize your socket and other resources for the Modbus server
int sock = create_server_socket(); // Function to create server socket
struct sockaddr_in addr;
socklen_t len = sizeof(addr);
while (1) {
int client_sock = accept(sock, (struct sockaddr*)&addr, &len);
if (client_sock == -1) { // Handle error or exception here
perror("Error accepting connection");
continue;
}
// Process new client connection
// Optionally add signal handling here to gracefully exit when needed
signal(SIGINT, handle_signal); // Example: Register SIGINT handler
// If you want to force a termination under certain conditions,
// consider using a thread with a global variable indicating the need to quit.
// Close the client socket after processing
close(client_sock);
}
return 0;
}
void handle_signal(int sig) {
switch(sig) {
case SIGINT:
printf("\nExiting program on request...\n");
// Implement cleanup steps before exiting
exit(0);
}
}
```
请注意,上述代码仅为示例性质,实际应用中可能需要根据具体的环境和需求调整错误处理、资源管理和信号处理的部分。对于`modbus_tcp_accept()`的具体使用细节以及相关的错误处理,应该参考Modbus库的文档或官方指南。
阅读全文
相关推荐
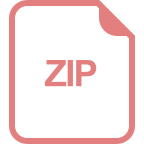
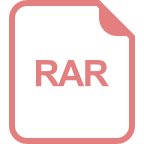
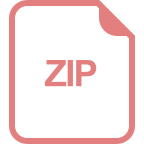
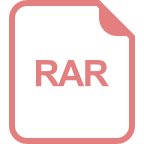
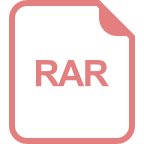

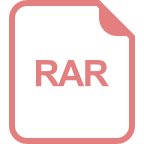
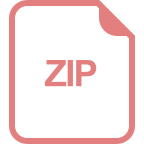
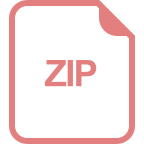
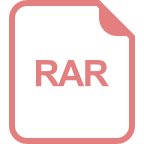
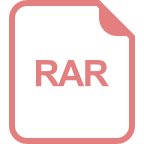
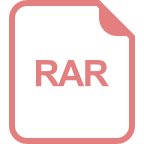
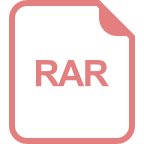
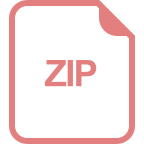