c++建立一个二叉搜索树,并进行查找算法的设计。
时间: 2024-12-15 08:23:49 浏览: 3
在C++中,我们可以创建一个二叉搜索树(Binary Search Tree,BST)的数据结构,它是一种特殊的二叉树,其中每个节点的值都大于左子树中所有节点的值,小于右子树中所有节点的值。以下是创建一个简单的二叉搜索树以及查找算法的基本设计:
```cpp
// 定义二叉搜索树结点
template <typename T>
class TreeNode {
public:
T val;
TreeNode* left;
TreeNode* right;
TreeNode(T x) : val(x), left(nullptr), right(nullptr) {}
};
// 插入操作
template <typename T>
TreeNode<T>* insert(TreeNode<T>* root, T val) {
if (root == nullptr) return new TreeNode<T>(val);
if (val < root->val) root->left = insert(root->left, val);
else root->right = insert(root->right, val);
return root;
}
// 查找操作
template <typename T>
TreeNode<T>* search(TreeNode<T>* root, T val) {
if (root == nullptr || root->val == val) return root;
if (val < root->val) return search(root->left, val);
return search(root->right, val);
}
// 示例
int main() {
// 创建一个空的二叉搜索树
TreeNode<int> *root = nullptr;
// 插入元素
root = insert(root, 50);
insert(root, 30);
insert(root, 20);
insert(root, 40);
insert(root, 70);
insert(root, 60);
// 查找元素
TreeNode<int>* found = search(root, 40); // 返回指向40的结点
if (found) {
std::cout << "Found element: " << found->val << std::endl;
} else {
std::cout << "Element not found." << std::endl;
}
return 0;
}
```
阅读全文
相关推荐




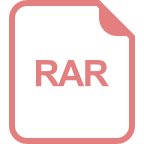












