net vb 如何在使用DrawImage保留图片的透明度
时间: 2024-09-23 07:07:33 浏览: 37
在.NET Framework的VB.NET中,如果你想要在使用`DrawImage`方法绘制图像时保留图片的透明度(也称为Alpha通道),你需要确保图片是以支持Alpha通道的格式存储,如PNG。以下是一个基本示例:
```vb
Imports System.Drawing.Drawing2D
' 假设你有一个支持Alpha通道的Bitmap对象 called myImage
Dim g As Graphics = Me.CreateGraphics() ' 创建一个图形对象用于绘图
Dim sourceRect As New Rectangle(0, 0, myImage.Width, myImage.Height) ' 图像源区域
g.DrawImage(myImage, DestinationRectangle, sourceRect, GraphicsUnit.Pixel, Color.White, 0, 0, sourceRect.Width, sourceRect.Height, GraphicsUnit.Pixel, Drawing2D.BlendMode.SourceAlpha) ' 传递BlendMode.SourceAlpha以保留透明度
' 确保清理图形上下文
g.Dispose()
```
这里的关键在于在`DrawImage`方法中最后一个参数,`BlendMode.SourceAlpha`表示采用源alpha模式,它会保持原图像的透明度。记得处理好各个矩形的位置和大小。
相关问题
vb操作png为透明背景图片的实例
### 回答1:
下面是一个将VB中的PNG图像操作为透明背景图片的示例:
```vb
Private Sub ConvertToTransparentBG()
' 加载PNG图像
Dim image As Image = Image.FromFile("D:\test.png")
' 创建一个具有透明背景的位图
Dim bitmap As New Bitmap(image.Width, image.Height, PixelFormat.Format32bppArgb)
' 设置位图的分辨率与图像一致
bitmap.SetResolution(image.HorizontalResolution, image.VerticalResolution)
' 创建图像绘制对象
Dim gfx As Graphics = Graphics.FromImage(bitmap)
' 清空位图并将其背景设置为透明
gfx.Clear(Color.Transparent)
' 在位图上绘制PNG图像
gfx.DrawImage(image, New Rectangle(0, 0, image.Width, image.Height))
' 保存处理后的图像为PNG文件(可以指定保存路径)
bitmap.Save("D:\transparent_bg.png", ImageFormat.Png)
' 清理资源
gfx.Dispose()
image.Dispose()
bitmap.Dispose()
End Sub
```
在示例中,我们首先加载PNG图像,并创建了一个具有透明背景的位图。然后,我们使用Graphics对象的DrawImage方法将加载的PNG图像绘制在位图上。最后,我们保存处理后的图像为PNG文件,并清理了使用到的资源。
### 回答2:
示例代码如下:
```vb
Imports System.Drawing
Imports System.Drawing.Imaging
Public Class Form1
Inherits Form
Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
Dim sourceImage As New Bitmap("input.png") ' 输入图片文件的路径
' 在内存中创建一个新的位图,并设置大小和原图一致
Dim targetImage As New Bitmap(sourceImage.Width, sourceImage.Height)
' 遍历原图的每一个像素
For y As Integer = 0 To sourceImage.Height - 1
For x As Integer = 0 To sourceImage.Width - 1
Dim pixel As Color = sourceImage.GetPixel(x, y) ' 获取当前像素的颜色
' 如果该像素的颜色是白色,则设置为透明
If pixel = Color.White Then
targetImage.SetPixel(x, y, Color.Transparent)
Else
targetImage.SetPixel(x, y, pixel) ' 否则保持原来的颜色
End If
Next
Next
targetImage.Save("output.png", ImageFormat.Png) ' 输出图片文件的路径
sourceImage.Dispose()
targetImage.Dispose()
End Sub
End Class
```
这个示例演示了使用VB.NET操作PNG图片,将白色像素设置为透明背景。首先,我们读取输入的PNG图片,并在内存中创建一个与原图大小相同的位图。然后,遍历原图的每一个像素,如果该像素的颜色是白色,则将其设置为透明;否则将保持原来的颜色。最后,将处理后的图像保存为一个新的PNG文件。
注意,你需要将"input.png"和"output.png"分别替换为你自己的输入和输出图片的路径。
### 回答3:
下面是一个使用VB操作PNG为透明背景图片的示例:
首先,确保你已经在计算机上安装了Visual Basic语言的开发环境,比如Visual Studio。然后按照以下步骤进行操作:
1. 创建一个新的Visual Basic项目。
2. 在项目中导入System.Drawing命名空间,以便能够使用图像处理相关的类和方法。
3. 从文件系统中加载一个PNG图像,可以使用Image类的Fromfile方法。
4. 将该图像对象的背景色调整为透明,可以使用Color类的FromArgb方法来指定透明度为0。
5. 将处理后的图像保存到指定的位置,可以使用Image类的Save方法。
以下是具体的示例代码:
```
Imports System.Drawing
Module Module1
Sub Main()
' 加载PNG图像
Dim imagePath As String = "C:\path\to\your\image.png"
Dim image As Image = Image.FromFile(imagePath)
' 将背景色调整为透明
Dim transparentColor As Color = Color.FromArgb(0, 255, 255, 255)
image.MakeTransparent(transparentColor)
' 保存处理后的图像
Dim outputPath As String = "C:\path\to\your\output.png"
image.Save(outputPath)
' 释放图像资源
image.Dispose()
End Sub
End Module
```
请确保替换`"C:\path\to\your\image.png"`和`"C:\path\to\your\output.png"`为你实际的文件路径。运行以上代码后,你将会得到一个具有透明背景的PNG图像。
希望这个例子对你有帮助!
阅读全文
相关推荐
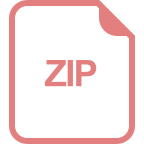
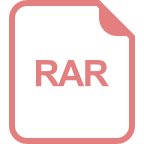
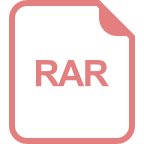
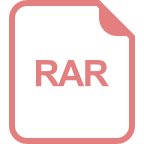
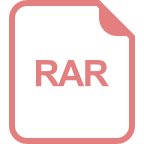
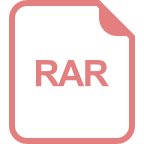
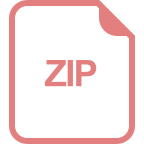
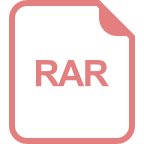
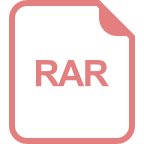
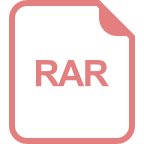
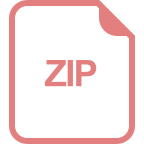
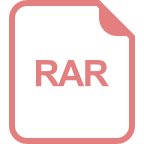
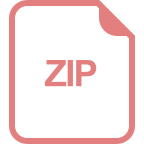
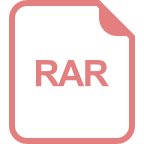
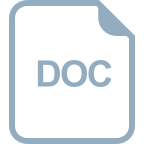
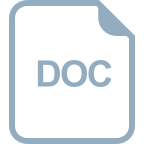