导出excel调整单元格宽度工具类 java
时间: 2024-12-18 10:12:10 浏览: 8
在Java中,创建一个用于导出Excel并自动调整单元格宽度的工具类,通常会利用Apache POI库,它是处理Microsoft Office格式文件如Excel的流行选择。以下是一个简单的示例,展示如何编写这样的工具类:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelWidthAdjuster {
private static final int AUTO_SIZE_COLUMN_WIDTH = -1; // 自动调整列宽的标记
public void exportToExcelWithAdjustedWidth(OutputStream outputStream, Workbook workbook) throws IOException {
try (FileOutputStream fos = new FileOutputStream(outputStream);
Sheet sheet = workbook.createSheet("Your Sheet")) {
// 获取工作表的第一行数据作为列标题
Row headerRow = sheet.createRow(0);
List<String> columnTitles = ... // 这里填充您的列标题列表
for (int i = 0; i < columnTitles.size(); i++) {
Cell cell = headerRow.createCell(i);
cell.setCellValue(columnTitles.get(i));
cell.setCellStyle(getAutoFitColumnStyle(sheet, i + 1)); // 设置自适应列宽
}
// 如果需要批量设置所有数据区域的列宽为自动大小
adjustAllColumnsWidth(sheet, AUTO_SIZE_COLUMN_WIDTH);
workbook.write(fos);
}
}
privateCellStyle getAutoFitColumnStyle(Sheet sheet, int columnIndex) {
return sheet.getRow((short) 0).getCell(columnIndex).getCellStyle().copy();
}
private void adjustAllColumnsWidth(Row row, int autoSizeColumnWidth) {
for (Cell cell : row) {
if (cell.getColumnIndex() != autoSizeColumnWidth) continue;
cell.setCellStyle(newCellStyle(autoSizeColumnWidth));
}
}
private staticCellStyle newCellStyle(int width) {
XSSFWorkbook wb = (XSSFWorkbook) wb;
return wb.createCellStyle()
.setWrapText(true)
.setHorizontallyCentered(true)
.setAlignment(HorizontalAlignment.CENTER)
.setColumnWidth(width);
}
}
// 使用示例
Workbook workbook = new XSSFWorkbook();
OutputStream outputStream = ... // 这里获取你的输出流
ExcelWidthAdjuster excelAdj = new ExcelWidthAdjuster();
excelAdj.exportToExcelWithAdjustedWidth(outputStream, workbook);
```
阅读全文
相关推荐
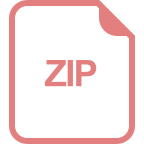
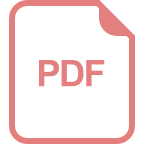
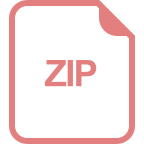















