函数设置形参表示数组C++
时间: 2024-10-18 09:10:25 浏览: 23
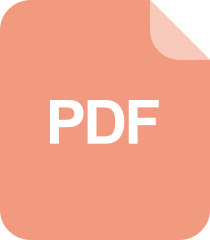
c++将数组名作为函数参数对数组元素进行相应的运算

在C++中,函数可以接受数组作为参数,通常通过指针或引用来间接处理。这是因为C++不直接支持传递多维数组,这是为了保持语言的高效性和内存管理。以下是两种常见的方法:
1. **通过指针**:
- 使用`T*`类型(T是你数组元素的类型),声明函数接收指向数组首元素的指针,例如:
```cpp
void printArray(int arr[], int size) {
for (int i = 0; i < size; ++i) {
cout << arr[i] << " ";
}
}
```
这样函数就可以遍历整个数组了。
2. **通过引用**:
- 使用`const T(&)`或`T(&)`,声明函数接收指向数组的引用,同样可以访问数组元素:
```cpp
void processArray(const int (&arr)[size]) { // 或者不加 const 如果需要修改数组内容
// ...
}
```
引用提供的是对原始数组的直接访问,不会创建额外的副本。
阅读全文
相关推荐
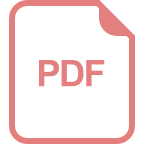
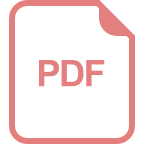

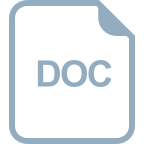
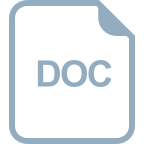











