connect toolButton 打开文件
时间: 2024-12-18 09:32:30 浏览: 4
在Qt中,如果你想要通过`toolButton`工具按钮来实现打开文件的功能,通常你会先将按钮的点击(click)信号(SIGNAL("clicked()"))连接到一个槽函数(SLOT),该槽函数负责具体的打开文件操作。这里是一个简单的示例:
```cpp
QObject::connect(toolButton, &QPushButton::clicked, this, [this]() {
QString fileName = QFileDialog::getOpenFileName(this, tr("选择文件"), "", tr("所有文件 (*);;文本文件 (*.txt)"));
if (!fileName.isEmpty()) {
// 这里是对选定文件进行后续处理,例如读取、保存等
qDebug() << "选择了文件:" << fileName;
}
});
```
在这个代码片段中,
- `QObject::connect()` 函数连接了信号(来自`toolButton`的`clicked()`信号)到槽函数(`this`对象中的lambda表达式)。
- lambda表达式内部调用了`QFileDialog::getOpenFileName()`来显示一个文件选择对话框,允许用户选择文件。
- 如果用户选择了一个文件,`fileName`变量存储了选中的文件名。
相关问题
connect toolButton 打开文件,文件路径显示在lineEdit
在Qt中,如果你想要通过`toolButton`按钮打开文件,并将文件路径显示在`lineEdit`控件中,你可以使用`QFileDialog`和槽函数来实现这个功能。以下是基本步骤:
1. 首先,在`.ui`设计文件中,你需要连接`toolButton`和`lineEdit`到相应的槽函数。
```xml
<!-- 在.ui文件里 -->
<QRadioButton>...</RadioButton>
<button name="toolButton" ...>选择文件</button>
<LineEdit name="lineEdit" ...></LineEdit>
<!-- 这里的信号槽连接 -->
< Connections >
<ActionTriggered name="toolButton_clicked">
<Slot>openFile(lineEdit)</Slot>
</ActionTriggered>
</Connections>
```
2. 然后在你的`.cpp`文件中,创建`openFile`函数并使用`QFileDialog`打开文件:
```cpp
#include "ui_form.h"
void YourClass::openFile(QLineEdit* lineEdit)
{
QString fileName = QFileDialog::getOpenFileName(this, tr("选择文件"), "", tr("所有文件(*);;文本文件 (*.txt)")); // 弹出文件选择框
if (!fileName.isEmpty()) { // 如果用户选择了文件
lineEdit->setText(fileName); // 将文件路径设置到lineEdit中
}
}
```
qt connect toolButton 打开文件,文件路径显示在lineEdit
在Qt中,你可以使用信号(Signal)和槽(Slot)机制来实现这样的功能。当你点击工具按钮(toolButton)时,你需要创建一个连接,使得这个按钮的点击事件触发一个打开文件的函数,并将选择的文件路径显示在LineEdit控件中。
首先,在`.ui`设计文件中,确保toolButton和lineEdit已经存在并且有相应的信号和槽声明。例如,假设`toolButton`有一个名为`clicked()`的信号,而`lineEdit`有一个用于接收路径的槽`setPath(path)`:
```ui
<button type="toolButton" name="toolButton" objectName="toolButton">
<property name="text">Open File</property>
</button>
<lineEdit name="lineEdit" objectName="lineEdit"/>
```
然后,在`.cpp`文件中,通过`QObject::connect()`函数来设置信号到槽的连接:
```cpp
#include "ui_form.h"
// ...
QObject::connect(toolButton, &QPushButton::clicked, this, &YourClass::openFile);
void YourClass::openFile() {
QString filePath = QFileDialog::getOpenFileName(nullptr, tr("Open File"), "", tr("All Files (*)"));
if (!filePath.isEmpty()) {
lineEdit->setText(filePath);
}
}
```
在这个例子中,`openFile()`方法会被当toolButton被点击时自动调用。它会弹出一个文件选择对话框让用户选择文件,然后把选择的文件路径设置到lineEdit里。
阅读全文
相关推荐
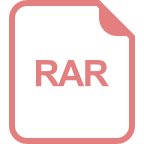
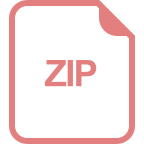
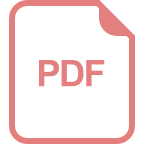


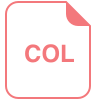


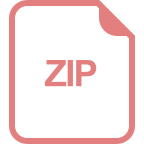
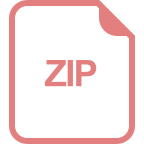