java写一个get请求 携带参数工具类 并往请求头里添加key值
时间: 2024-09-11 12:08:02 浏览: 40
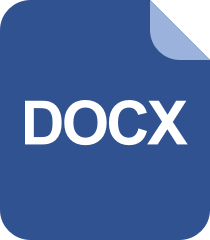
Https请求工具类,Java实现http请求,https请求
在Java中,你可以创建一个工具类来简化GET请求的过程,同时携带参数并设置请求头。这里是一个简单的示例,我们将使用HttpURLConnection来进行HTTP请求:
首先,定义一个工具类,比如`RestRequestUtils.java`:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class RestRequestUtils {
public static String get(String url, Map<String, String> params, String apiKey) throws Exception {
StringBuilder query = new StringBuilder();
for (Map.Entry<String, String> entry : params.entrySet()) {
if (query.length() > 0) {
query.append("&");
}
query.append(entry.getKey()).append("=").append(entry.getValue());
}
// URL with parameters
URL obj = new URL(url + "?" + query.toString());
// Adding request header
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
con.setRequestProperty("Authorization", "Bearer " + apiKey); // Assuming 'Bearer' is the key for your API key
int responseCode = con.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return response.toString();
} else {
throw new RuntimeException("Failed : HTTP error code : " + responseCode);
}
}
}
```
在这个例子中,我们首先将传递的参数转换为URL查询字符串,然后通过`HttpURLConnection`发送GET请求,并在请求头中添加API密钥。如果服务器返回状态码为200(HTTP_OK),则读取响应内容并返回。
使用这个工具类的样例:
```java
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
try {
Map<String, String> params = new HashMap<>();
params.put("param1", "value1");
params.put("param2", "value2");
String apiKey = "your_api_key_here";
String result = RestRequestUtils.get("http://example.com/api", params, apiKey);
System.out.println(result);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
阅读全文
相关推荐
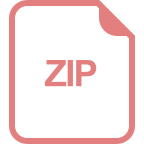
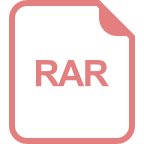
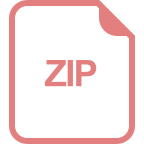
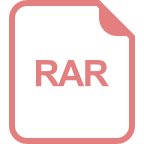
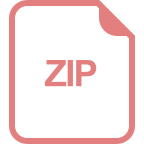
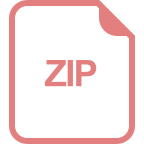
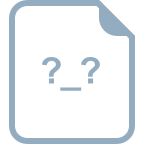
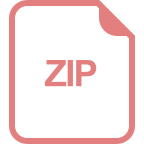
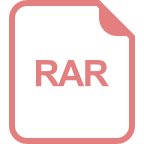
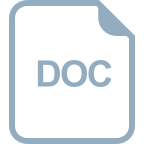