差分形式的牛顿插值c++代码
时间: 2024-09-30 16:00:50 浏览: 31
差分形式的牛顿插值,也称为高德插值法,是一种数值分析技术,用于计算给定数据点上的一次多项式近似。在C++中,你可以通过创建一个线性递归函数来实现。下面是一个简单的示例:
```cpp
#include <iostream>
#include <vector>
// 定义节点结构
struct Node {
double x;
double y;
};
// 计算差分
double diff(Node p, Node q) {
return q.y - p.y;
}
// 插值函数
double newton_interpolation(std::vector<Node>& points, int n) {
if (n == 0) {
return points[0].y; // 如果只有一个点,返回该点的y值
} else {
Node mid = {points[n-1].x + points[n-2].x, 0};
for (int i = 0; i < n; ++i) {
mid.y += diff(points[i], points[(i+1)%n]) / factorial(n);
}
return mid.y;
}
}
// 计算阶乘
unsigned long long factorial(int n) {
unsigned long long fact = 1;
for (int i = 2; i <= n; ++i) {
fact *= i;
}
return fact;
}
int main() {
std::vector<Node> points = {{0, 2}, {1, 5}, {2, 8}};
int n = points.size();
double interpolated_value = newton_interpolation(points, n);
std::cout << "Interpolated value at x=1.5 is: " << interpolated_value << std::endl;
return 0;
}
```
在这个例子中,我们首先定义了一个`Node`结构,然后实现了计算差分`diff()`和差分形式的牛顿插值`newton_interpolation()`函数。`main()`函数展示了如何使用这些函数对一组数据点进行插值。
阅读全文
相关推荐
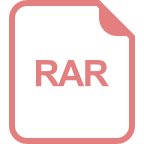
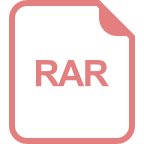
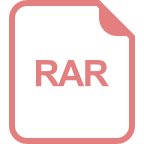
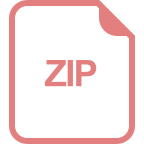
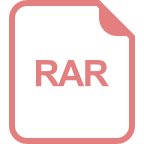
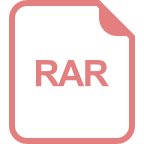
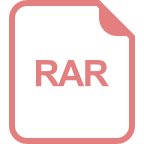
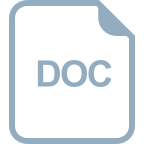
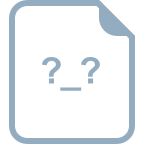
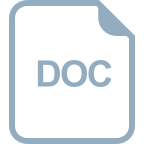
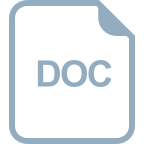
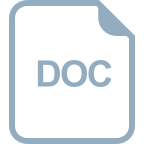
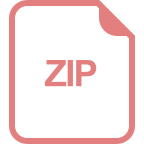