c http json
时间: 2023-08-14 15:30:53 浏览: 40
您需要对应用程序使用HTTP通信并使用JSON格式进行数据交换。下面是一个使用C语言进行HTTP请求并解析JSON响应的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <curl/curl.h>
#include <jansson.h>
int main(void)
{
CURL *curl;
CURLcode res;
char *url = "http://example.com/api";
char *post_data = "{\"key\": \"value\"}";
curl = curl_easy_init();
if (curl) {
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "Content-Type: application/json");
curl_easy_setopt(curl, CURLOPT_URL, url);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, post_data);
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
res = curl_easy_perform(curl);
if (res != CURLE_OK) {
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
} else {
long http_code = 0;
curl_easy_getinfo(curl, CURLINFO_RESPONSE_CODE, &http_code);
printf("HTTP Status Code: %ld\n", http_code);
json_error_t error;
json_t *root = json_loads(post_data, 0, &error);
if (!root) {
fprintf(stderr, "json_loads() failed: %s\n", error.text);
} else {
json_t *result = NULL;
json_object_get(root, "result", &result);
if (result) {
const char *result_str = json_string_value(result);
printf("Result: %s\n", result_str);
} else {
fprintf(stderr, "Failed to get 'result' field from JSON response.\n");
}
json_decref(root);
}
}
curl_slist_free_all(headers);
curl_easy_cleanup(curl);
}
return 0;
}
```
这个示例使用了`curl`库来进行HTTP请求,使用`jansson`库来解析JSON响应。您需要安装这两个库才能编译和运行示例代码。
相关推荐
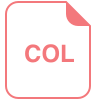
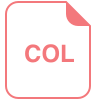
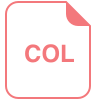
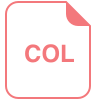
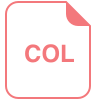









