java 同态加密代码
时间: 2023-12-08 17:05:14 浏览: 176
以下是使用 Paillier 同态加密算法实现的 Java 代码示例:
```java
import java.math.BigInteger;
import java.security.SecureRandom;
public class Paillier {
private final int CERTAINTY = 64;
private final SecureRandom random = new SecureRandom();
private BigInteger p;
private BigInteger q;
private BigInteger lambda;
private BigInteger n;
private BigInteger nsquare;
private BigInteger g;
public Paillier(int numBits) {
generateKeys(numBits);
}
public Paillier(BigInteger p, BigInteger q) {
setKeys(p, q);
}
public void generateKeys(int numBits) {
p = BigInteger.probablePrime(numBits / 2, random);
q = BigInteger.probablePrime(numBits / 2, random);
n = p.multiply(q);
nsquare = n.multiply(n);
g = n.add(BigInteger.ONE);
lambda = p.subtract(BigInteger.ONE).multiply(q.subtract(BigInteger.ONE)).divide(
p.subtract(BigInteger.ONE).gcd(q.subtract(BigInteger.ONE)));
}
public void setKeys(BigInteger p, BigInteger q) {
this.p = p;
this.q = q;
n = p.multiply(q);
nsquare = n.multiply(n);
g = n.add(BigInteger.ONE);
lambda = p.subtract(BigInteger.ONE).multiply(q.subtract(BigInteger.ONE)).divide(
p.subtract(BigInteger.ONE).gcd(q.subtract(BigInteger.ONE)));
}
public BigInteger encrypt(BigInteger m) {
BigInteger r = new BigInteger(n.bitLength(), random);
return g.modPow(m, nsquare).multiply(r.modPow(n, nsquare)).mod(nsquare);
}
public BigInteger add(BigInteger em1, BigInteger em2) {
return em1.multiply(em2).mod(nsquare);
}
public BigInteger multiply(BigInteger em, int k) {
return em.modPow(BigInteger.valueOf(k), nsquare);
}
public BigInteger decrypt(BigInteger c) {
BigInteger u = g.modPow(lambda, nsquare).subtract(BigInteger.ONE).divide(n).modInverse(n);
return c.modPow(lambda, nsquare).subtract(BigInteger.ONE).divide(n).multiply(u).mod(n);
}
}
```
使用示例:
```java
Paillier paillier = new Paillier(1024);
BigInteger m1 = BigInteger.valueOf(42);
BigInteger m2 = BigInteger.valueOf(123);
BigInteger em1 = paillier.encrypt(m1);
BigInteger em2 = paillier.encrypt(m2);
BigInteger em3 = paillier.add(em1, em2);
BigInteger em4 = paillier.multiply(em1,5);
BigInteger dm3 = paillier.decrypt(em3);
BigInteger dm4 = paillier.decrypt(em4);
System.out.println(dm3); // output: 165
System.out.println(dm4); // output: 210
```
阅读全文
相关推荐
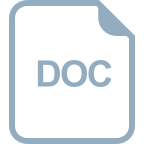
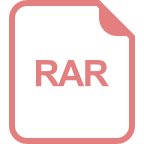
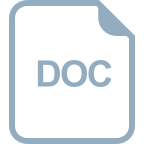

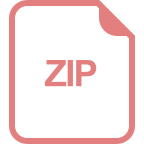
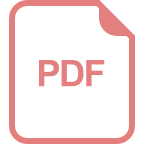
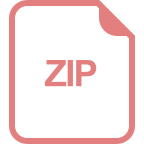
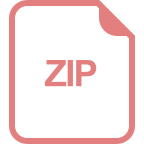
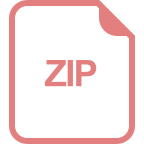
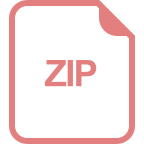
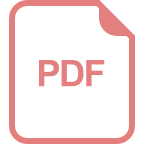
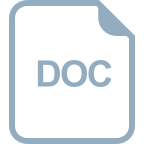
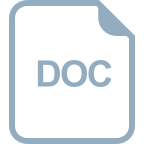
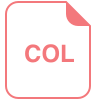