print(“requests:”,res.text)
时间: 2024-06-09 11:09:07 浏览: 5
这段代码是用来打印出来 HTTP 请求的响应结果,其中 res.text 是响应结果的文本内容。通常在使用 Python 编写 Web 爬虫时,我们会用到 requests 库来发送 HTTP 请求,并通过 res.text 属性来获取响应结果的文本内容。例如,如果我们向某个网站发送了一个 GET 请求,那么可以通过以下代码来获取响应结果的文本内容:
```python
import requests
url = 'http://www.example.com'
res = requests.get(url)
print('requests:', res.text)
```
这样就可以将响应结果打印出来了。当然,如果响应结果是 JSON 格式的,我们还可以通过 res.json() 方法来将其转换为 Python 对象。
相关问题
import requests import os from bs4 import BeautifulSoup class book_spider(): def __init__(self,root_url): self.root_url=root_url self.book_list=[] #一级页面中获取的数据(二级页面地址)存放于此列表 self.chapter_list=[] #二级页面中获取的数据(三级页面地址和章节名)存放于此列表 def get_url(url): while True: try: res=requests.get(url) if res.status_code==200: res.encoding =res.apparent_encoding print("页面获取成功") return res.text else: print("页面返回异常",res.status_code) except: print("页面获取错误") def get_book_list(self.url): res = self.get_url(url) html = BeautifulSoup(res,"html.parser") a_list = html.find_all("a",{"class":"name"}) for a in a_list: self.book_list.append(a["href"]) self.book_list = [self.root_url+i for i in self.bbok_list] self.book_list.remove('http://10.1.88.252:7000/庆余年') print(book_list) def get_chapter_list(self,url): res = self.get_url(url) html = BeautifulSoup(res,"html.parser") a_list = html.find_all("a",{"class":"chapter"}) for a in a_list: self.chapter_list.append((a["href"],a.text.replace("\n",""))) def get_content(self.chapter): url = self.root_url + chapter[0] print(url) book_name = chapter[0].split("/")[1] print(book_name) if not os.path.exists(book_name): os.mkdir(book_name) res = self.get_url(url) html = BeautifulSoup(res,"html.parser") content = html.find("div",{"id":"content"}).text print(content) path = os.path.join(book_name,chapter[1]) with open(path,"w",encoding="utf8") as f: f.write(content) def main(): self.get_book_list(self.root_url) for book in self.book_list: self.get_chapter_liat(book) for chapter in chapter_list: self.get_content(chapter) book_s = book_spider("http://10.1.88.252:7000") book_s.main()这是一段爬虫代码,找出里面的错误并改正
有以下几个错误:
1. 在 `get_url` 方法中,需要在 `self` 参数之前添加 `@staticmethod` 装饰器,使其成为静态方法。
2. 在 `get_book_list` 方法中,`print(book_list)` 应该改为 `print(self.book_list)`,因为 `book_list` 是类成员变量。
3. 在 `get_book_list` 方法中,将 `self.bbok_list` 改为 `self.book_list`。
4. 在 `get_chapter_list` 方法中,将 `self.chapter_list.append((a["href"],a.text.replace("\n","")))` 改为 `self.chapter_list.append((self.root_url+a["href"],a.text.replace("\n","")))`,因为需要获取完整的章节链接。
5. 在 `get_content` 方法中,将 `print(url)` 和 `print(book_name)` 改为注释,因为这些是调试信息。
改正后的代码如下:
```
import requests
import os
from bs4 import BeautifulSoup
class book_spider():
def __init__(self,root_url):
self.root_url=root_url
self.book_list=[]
self.chapter_list=[]
@staticmethod
def get_url(url):
while True:
try:
res=requests.get(url)
if res.status_code==200:
res.encoding =res.apparent_encoding
print("页面获取成功")
return res.text
else:
print("页面返回异常",res.status_code)
except:
print("页面获取错误")
def get_book_list(self,url):
res = self.get_url(url)
html = BeautifulSoup(res,"html.parser")
a_list = html.find_all("a",{"class":"name"})
for a in a_list:
self.book_list.append(self.root_url + a["href"])
self.book_list.remove('http://10.1.88.252:7000/庆余年')
print(self.book_list)
def get_chapter_list(self,url):
res = self.get_url(url)
html = BeautifulSoup(res,"html.parser")
a_list = html.find_all("a",{"class":"chapter"})
for a in a_list:
self.chapter_list.append((self.root_url+a["href"],a.text.replace("\n","")))
def get_content(self,chapter):
url = chapter[0]
book_name = chapter[0].split("/")[1]
if not os.path.exists(book_name):
os.mkdir(book_name)
res = self.get_url(url)
html = BeautifulSoup(res,"html.parser")
content = html.find("div",{"id":"content"}).text
path = os.path.join(book_name,chapter[1])
with open(path,"w",encoding="utf8") as f:
f.write(content)
def main(self):
self.get_book_list(self.root_url)
for book in self.book_list:
self.get_chapter_list(book)
for chapter in self.chapter_list:
self.get_content(chapter)
book_s = book_spider("http://10.1.88.252:7000")
book_s.main()
```
帮我把一下代码设置一个合理请求头,并加入一个延时import requests import os from bs4 import BeautifulSoup class NovelDownloader: def __init__(self, root_url): self.root_url = root_url self.book_list = [] self.chapter_list = [] def get_url(self, url): while True: try: res = requests.get(url) if res.status_code == 200: print("页面获取成功!") return res.text else: print("页面返回异常!", res.status_code) except: print("页面获取错误!") def get_book_list(self): res = self.get_url(self.root_url) html = BeautifulSoup(res, "html.parser") a_list = html.find_all("a", {"class": "name"}) for a in a_list: self.book_list.append(a["href"]) self.book_list = [self.root_url + i for i in self.book_list] self.book_list.remove('http://www.biquge5200.cc/') def get_chapter_list(self, url): res = self.get_url(url) html = BeautifulSoup(res, "html.parser") a_list = html.find_all("a", {"class": "chapter"}) for a in a_list: self.chapter_list.append((a["href"], a.text.replace("\n", ""))) def get_content(self, chapter): url = self.root_url + chapter[0] print(url) book_name = chapter[0].split("/")[1] print(book_name) if not os.path.exists(book_name): os.mkdir(book_name) res = self.get_url(url) html = BeautifulSoup(res, "html.parser") content = html.find("div", {"id": "content"}).text print(content) path = os.path.join(book_name, chapter[1]) with open(path, "w", encoding="utf8") as f: f.write(content) def main(self): self.get_book_list() for book in self.book_list: self.get_chapter_list(book) for chapter in self.chapter_list: self.get_content(chapter) if __name__ == '__main__': root_url = "http://www.biquge5200.cc/" nd = NovelDownloader(root_url) nd.main()
好的,我可以帮你添加一个合理的请求头和延时。在 `get_url` 方法中,你可以添加一个请求头字典,来模拟浏览器发送请求。同时,在每次请求后添加一个时间延时,避免频繁请求被网站屏蔽。
修改后的代码如下所示:
```python
import requests
import os
import time
from bs4 import BeautifulSoup
class NovelDownloader:
def __init__(self, root_url):
self.root_url = root_url
self.book_list = []
self.chapter_list = []
self.headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"
} # 添加请求头
def get_url(self, url):
while True:
try:
res = requests.get(url, headers=self.headers)
if res.status_code == 200:
print("页面获取成功!")
return res.text
else:
print("页面返回异常!", res.status_code)
except:
print("页面获取错误!")
time.sleep(1) # 添加延时
def get_book_list(self):
res = self.get_url(self.root_url)
html = BeautifulSoup(res, "html.parser")
a_list = html.find_all("a", {"class": "name"})
for a in a_list:
self.book_list.append(a["href"])
self.book_list = [self.root_url + i for i in self.book_list]
self.book_list.remove('http://www.biquge5200.cc/')
def get_chapter_list(self, url):
res = self.get_url(url)
html = BeautifulSoup(res, "html.parser")
a_list = html.find_all("a", {"class": "chapter"})
for a in a_list:
self.chapter_list.append((a["href"], a.text.replace("\n", "")))
def get_content(self, chapter):
url = self.root_url + chapter[0]
print(url)
book_name = chapter[0].split("/")[1]
print(book_name)
if not os.path.exists(book_name):
os.mkdir(book_name)
res = self.get_url(url)
html = BeautifulSoup(res, "html.parser")
content = html.find("div", {"id": "content"}).text
print(content)
path = os.path.join(book_name, chapter[1])
with open(path, "w", encoding="utf8") as f:
f.write(content)
def main(self):
self.get_book_list()
for book in self.book_list:
self.get_chapter_list(book)
for chapter in self.chapter_list:
self.get_content(chapter)
if __name__ == '__main__':
root_url = "http://www.biquge5200.cc/"
nd = NovelDownloader(root_url)
nd.main()
```
这样就可以更好地模拟浏览器发送请求,避免被网站屏蔽。另外,可以根据需要调整延时时间,以达到更好的效果。
相关推荐
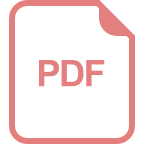
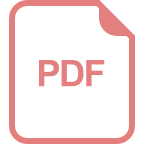




import requests from bs4 import BeautifulSoup import lxml headers = { "User-Agent": "Mozilla/5.0 (Macintosh; Intel)" } for start_name in range(0, 250, 25): res = requests.get(f"https://book.douban.com/top250?start=", headers=headers) print(res.status_code) soup = BeautifulSoup(res.text, 'lxml') t1 = soup.findAll('div', attrs={'class': 'pl2'}) for i in t1: t2 = i.find('a').text.strip() # 去除标签中的空格 print(t2);删除':'前的空白



import requests from bs4 import BeautifulSoup import lxml headers = { "User-Agent": "Mozilla/5.0 (Macintosh; Intel)" } for start_name in range(0, 250, 25): res = requests.get(f"https://book.douban.com/top250?start=", headers=headers) print(res.status_code) soup = BeautifulSoup(res.text, 'lxml') t1 = soup.findAll('div', attrs={'class': 'pl2'}) for i in t1: t2 = i.find('a') print(t2) break;去除输出中的空格






