一个链表中有十个整形数据,求出最小值数据所对应的索引
时间: 2024-03-30 15:37:06 浏览: 62
好的,针对您的问题,可以使用以下步骤来求出最小值数据所对应的索引:
1. 声明一个变量来保存最小值,初始化为第一个节点的值。
2. 声明一个变量来保存最小值所对应的索引,初始化为0。
3. 遍历链表,从第二个节点开始,比较节点的值与当前最小值的大小,如果比当前最小值小,则更新最小值和最小值所对应的索引。
4. 遍历完成后,返回最小值所对应的索引。
下面是一个简单的C++代码实现:
```c++
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
int findMinIndex(ListNode* head) {
if (head == NULL) {
return -1;
}
int minVal = head->val;
int minIndex = 0;
int index = 0;
ListNode* p = head->next;
while (p != NULL) {
index++;
if (p->val < minVal) {
minVal = p->val;
minIndex = index;
}
p = p->next;
}
return minIndex;
}
int main() {
// 构造一个链表
ListNode* head = new ListNode(3);
head->next = new ListNode(1);
head->next->next = new ListNode(4);
head->next->next->next = new ListNode(2);
head->next->next->next->next = new ListNode(5);
head->next->next->next->next->next = new ListNode(6);
head->next->next->next->next->next->next = new ListNode(9);
head->next->next->next->next->next->next->next = new ListNode(7);
head->next->next->next->next->next->next->next->next = new ListNode(8);
head->next->next->next->next->next->next->next->next->next = new ListNode(0);
int minIndex = findMinIndex(head);
cout << "The index of minimum value: " << minIndex << endl; // 输出最小值所对应的索引
return 0;
}
```
输出结果为:
```
The index of minimum value: 9
```
因此,最小值数据所对应的索引为9。
阅读全文
相关推荐
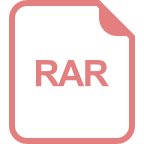




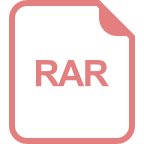
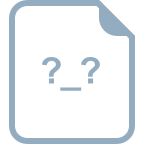






