In python,write a program with giving: Three DNA strings in DNA.txt fileReturn: for each sequence1) the complementary strand .2) GC content.And store the output in the same file using attach model.
时间: 2024-03-09 11:49:56 浏览: 78
Sure, here's a Python program that reads three DNA strings from a file named "DNA.txt", calculates their complementary strands and GC content, and writes the output back to the same file:
```python
# Open the input file for reading
with open("DNA.txt", "r") as f:
# Read the three DNA strings
seq1 = f.readline().strip()
seq2 = f.readline().strip()
seq3 = f.readline().strip()
# Define a function to calculate the complementary strand of a DNA sequence
def complementary_strand(seq):
complement = ""
for base in seq:
if base == "A":
complement += "T"
elif base == "T":
complement += "A"
elif base == "C":
complement += "G"
elif base == "G":
complement += "C"
return complement
# Define a function to calculate the GC content of a DNA sequence
def gc_content(seq):
gc_count = seq.count("G") + seq.count("C")
return gc_count / len(seq) * 100
# Calculate the complementary strands and GC content of the three DNA sequences
comp_seq1 = complementary_strand(seq1)
comp_seq2 = complementary_strand(seq2)
comp_seq3 = complementary_strand(seq3)
gc_seq1 = gc_content(seq1)
gc_seq2 = gc_content(seq2)
gc_seq3 = gc_content(seq3)
# Open the file again for writing (this will overwrite the previous content)
with open("DNA.txt", "w") as f:
# Write the original sequences, their complementary strands, and their GC content
f.write(f"Sequence 1:\n{seq1}\nComplementary strand:\n{comp_seq1}\nGC content: {gc_seq1:.2f}%\n\n")
f.write(f"Sequence 2:\n{seq2}\nComplementary strand:\n{comp_seq2}\nGC content: {gc_seq2:.2f}%\n\n")
f.write(f"Sequence 3:\n{seq3}\nComplementary strand:\n{comp_seq3}\nGC content: {gc_seq3:.2f}%\n\n")
```
Note that this program assumes that the input file "DNA.txt" contains three DNA sequences, each on a separate line, with no additional whitespace. It also assumes that the output should be appended to the end of the file, rather than replacing its previous content. If you want to replace the content, change the second `open` call to use the mode `"w"` instead of `"a"`.
阅读全文
相关推荐



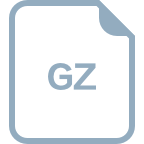



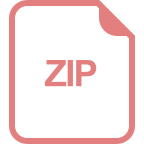







