linux4.9下如何编写应用程序控制I2C
时间: 2024-05-01 15:22:51 浏览: 128
在Linux 4.9下,可以使用i2c-dev驱动程序来控制I2C总线。以下是一些基本步骤:
1. 安装i2c-tools: `sudo apt-get install i2c-tools`
2. 确认I2C总线是否已启用:`sudo i2cdetect -l`
3. 打开I2C总线设备文件:`sudo modprobe i2c-dev`,这会在/dev目录下创建i2c-N文件,其中N是总线号。
4. 用open()函数打开设备文件,使用ioctl()函数发送I2C信号。
以下是一些示例代码,用于向I2C设备写入和读取数据:
```c
#include <stdio.h>
#include <fcntl.h>
#include <linux/i2c-dev.h>
#include <unistd.h>
int main()
{
int file;
char *filename = "/dev/i2c-1"; // I2C总线1
int addr = 0x68; // I2C从设备地址
char buf[2];
if ((file = open(filename, O_RDWR)) < 0) {
printf("Failed to open I2C device.\n");
return 1;
}
if (ioctl(file, I2C_SLAVE, addr) < 0) {
printf("Failed to select I2C device.\n");
return 1;
}
// 写入数据
buf[0] = 0x00; // 寄存器地址
buf[1] = 0x12; // 数据
if (write(file, buf, 2) != 2) {
printf("Failed to write to I2C device.\n");
return 1;
}
// 读取数据
buf[0] = 0x00; // 寄存器地址
if (write(file, buf, 1) != 1) {
printf("Failed to write to I2C device.\n");
return 1;
}
if (read(file, buf, 1) != 1) {
printf("Failed to read from I2C device.\n");
return 1;
}
printf("Data read: 0x%x\n", buf[0]);
close(file);
return 0;
}
```
在以上示例代码中,我们使用了/dev/i2c-1作为I2C总线设备文件,选择了从设备地址0x68,写入了0x12到寄存器地址0x00,并读取了该地址的数据。如果一切正常,将会输出读取的数据。
阅读全文
相关推荐
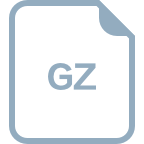
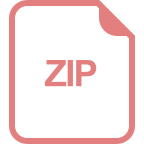
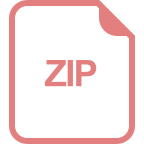
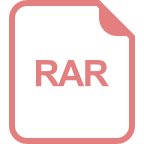
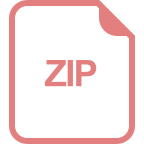
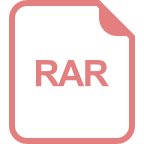
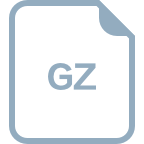
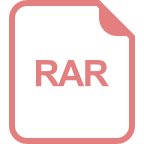
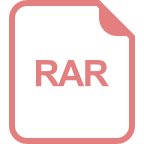
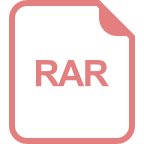
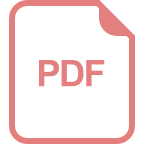
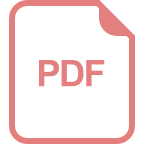
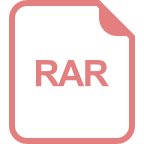
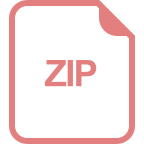