用指向指针的指针的方法对n个字符串排序并输出。要求将排序单独写成一个函数,n和个整数在主函数中输入。最后在主函数中输出。用c语言来写
时间: 2024-03-10 20:47:28 浏览: 17
好的,下面是排序函数的代码实现,使用指向指针的指针的方法进行排序:
```c
void sort(char **str, int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (strcmp(str[j], str[j+1]) > 0) {
char *temp = str[j];
str[j] = str[j+1];
str[j+1] = temp;
}
}
}
}
```
主函数中,我们可以先定义n个字符串的指针数组,然后在输入n个字符串时,将每个字符串的指针存储到指针数组中。最后调用排序函数对指针数组进行排序,再依次输出排序后的字符串即可。
```c
#include <stdio.h>
#include <string.h>
void sort(char **str, int n);
int main() {
int n;
printf("请输入字符串个数: ");
scanf("%d", &n);
char *str[n];
printf("请输入%d个字符串:\n", n);
for (int i = 0; i < n; i++) {
char s[100];
scanf("%s", s);
str[i] = (char *)malloc(strlen(s) + 1);
strcpy(str[i], s);
}
sort(str, n);
printf("排序后的字符串:\n");
for (int i = 0; i < n; i++) {
printf("%s\n", str[i]);
free(str[i]);
}
return 0;
}
void sort(char **str, int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (strcmp(str[j], str[j+1]) > 0) {
char *temp = str[j];
str[j] = str[j+1];
str[j+1] = temp;
}
}
}
}
```
注意,在排序结束后,需要释放每个字符串的内存空间,避免内存泄漏。
相关推荐
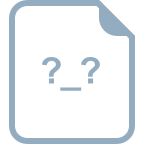
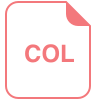
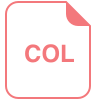
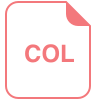
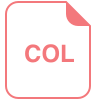
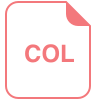









