pycharm用pygame写大球吃小球
时间: 2023-07-24 22:32:49 浏览: 133
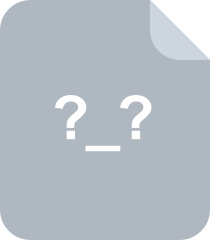
pygame大球吃小球
好的,我可以给你一个简单的示例——大球吃小球的游戏。
首先,我们需要安装Pygame库。在PyCharm中,可以打开终端并输入以下命令来安装Pygame:
```
pip install pygame
```
然后,我们可以创建一个窗口并在窗口中绘制大球和小球。可以使用Pygame中的Surface和draw模块来实现:
```python
import pygame
import sys
import random
pygame.init()
# 设置窗口大小和标题
size = width, height = 640, 480
screen = pygame.display.set_mode(size)
pygame.display.set_caption('大球吃小球')
# 设置大球的初始位置和速度
big_ball_pos = [width // 2, height // 2]
big_ball_speed = [0, 0]
# 设置小球的数量和半径
num_balls = 10
min_radius = 10
max_radius = 30
# 生成小球的位置和速度
balls = []
for i in range(num_balls):
radius = random.randint(min_radius, max_radius)
x = random.randint(radius, width - radius)
y = random.randint(radius, height - radius)
speed = [random.randint(-5, 5), random.randint(-5, 5)]
balls.append([x, y, speed, radius])
# 设置大球的半径和颜色
big_ball_radius = 50
big_ball_color = (255, 0, 0)
# 设置小球的颜色
ball_color = (0, 0, 255)
# 进入游戏循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
# 让大球移动
big_ball_pos[0] += big_ball_speed[0]
big_ball_pos[1] += big_ball_speed[1]
# 判断大球是否碰到边界
if big_ball_pos[0] < big_ball_radius or big_ball_pos[0] > width - big_ball_radius:
big_ball_speed[0] = -big_ball_speed[0]
if big_ball_pos[1] < big_ball_radius or big_ball_pos[1] > height - big_ball_radius:
big_ball_speed[1] = -big_ball_speed[1]
# 绘制大球和小球
screen.fill((255, 255, 255)) # 设置背景色为白色
pygame.draw.circle(screen, big_ball_color, big_ball_pos, big_ball_radius)
for i in range(num_balls):
x, y, speed, radius = balls[i]
x += speed[0]
y += speed[1]
# 判断小球是否碰到边界
if x < radius or x > width - radius:
speed[0] = -speed[0]
if y < radius or y > height - radius:
speed[1] = -speed[1]
# 判断大球是否吃掉了小球
if (x - big_ball_pos[0]) ** 2 + (y - big_ball_pos[1]) ** 2 <= big_ball_radius ** 2:
big_ball_radius += int(radius / 10)
balls.pop(i)
num_balls -= 1
break
balls[i] = [x, y, speed, radius]
pygame.draw.circle(screen, ball_color, (x, y), radius)
pygame.display.update() # 更新窗口
```
最后,我们可以将以上代码整合在一起,并在PyCharm中运行:
```python
import pygame
import sys
import random
pygame.init()
size = width, height = 640, 480
screen = pygame.display.set_mode(size)
pygame.display.set_caption('大球吃小球')
big_ball_pos = [width // 2, height // 2]
big_ball_speed = [0, 0]
num_balls = 10
min_radius = 10
max_radius = 30
balls = []
for i in range(num_balls):
radius = random.randint(min_radius, max_radius)
x = random.randint(radius, width - radius)
y = random.randint(radius, height - radius)
speed = [random.randint(-5, 5), random.randint(-5, 5)]
balls.append([x, y, speed, radius])
big_ball_radius = 50
big_ball_color = (255, 0, 0)
ball_color = (0, 0, 255)
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
big_ball_pos[0] += big_ball_speed[0]
big_ball_pos[1] += big_ball_speed[1]
if big_ball_pos[0] < big_ball_radius or big_ball_pos[0] > width - big_ball_radius:
big_ball_speed[0] = -big_ball_speed[0]
if big_ball_pos[1] < big_ball_radius or big_ball_pos[1] > height - big_ball_radius:
big_ball_speed[1] = -big_ball_speed[1]
screen.fill((255, 255, 255))
pygame.draw.circle(screen, big_ball_color, big_ball_pos, big_ball_radius)
for i in range(num_balls):
x, y, speed, radius = balls[i]
x += speed[0]
y += speed[1]
if x < radius or x > width - radius:
speed[0] = -speed[0]
if y < radius or y > height - radius:
speed[1] = -speed[1]
if (x - big_ball_pos[0]) ** 2 + (y - big_ball_pos[1]) ** 2 <= big_ball_radius ** 2:
big_ball_radius += int(radius / 10)
balls.pop(i)
num_balls -= 1
break
balls[i] = [x, y, speed, radius]
pygame.draw.circle(screen, ball_color, (x, y), radius)
pygame.display.update()
```
运行后,程序会创建一个窗口并在窗口中绘制一个大球和多个小球,大球可以吃掉小球。按下窗口的关闭按钮或者按下Ctrl+C可以退出程序。
阅读全文
相关推荐
















