在mfc中,写一个CDC类做分图层绘图的例子。
时间: 2023-05-26 13:01:50 浏览: 297
以下是一个简单的例子,使用CDC类实现分图层绘图:
首先,创建一个MFC应用程序,并在主框架窗口类(CMainFrame)中添加以下代码:
// CMainFrame.h
class CMainFrame : public CFrameWnd
{
public:
CMainFrame();
protected:
// 重写
virtual BOOL OnCreateClient(LPCREATESTRUCT lpcs, CCreateContext* pContext);
// 声明三个CDC指针,分别用于绘制三个图层
CDC* m_pdcLayer1;
CDC* m_pdcLayer2;
CDC* m_pdcLayer3;
// 定义三个矩形,作为三个图层的区域
CRect m_rectLayer1;
CRect m_rectLayer2;
CRect m_rectLayer3;
// 实现绘制函数
void DrawLayers();
};
// CMainFrame.cpp
CMainFrame::CMainFrame()
{
// 定义三个区域,各自占据整个客户区,并进行分割
GetClientRect(m_rectLayer1);
m_rectLayer2 = m_rectLayer1;
m_rectLayer3 = m_rectLayer1;
m_rectLayer1.right /= 3;
m_rectLayer2.left = m_rectLayer1.right;
m_rectLayer2.right = m_rectLayer1.right * 2;
m_rectLayer3.left = m_rectLayer2.right;
// 创建CDC对象,并关联到三个指针上
m_pdcLayer1 = new CDC();
m_pdcLayer1->CreateCompatibleDC(NULL);
m_pdcLayer2 = new CDC();
m_pdcLayer2->CreateCompatibleDC(NULL);
m_pdcLayer3 = new CDC();
m_pdcLayer3->CreateCompatibleDC(NULL);
// 创建三个位图,并关联到CDC对象上
m_pdcLayer1->CreateCompatibleBitmap(GetDC(), m_rectLayer1.Width(), m_rectLayer1.Height());
m_pdcLayer1->SelectObject(m_pdcLayer1->GetCurrentBitmap());
m_pdcLayer2->CreateCompatibleBitmap(GetDC(), m_rectLayer2.Width(), m_rectLayer2.Height());
m_pdcLayer2->SelectObject(m_pdcLayer2->GetCurrentBitmap());
m_pdcLayer3->CreateCompatibleBitmap(GetDC(), m_rectLayer3.Width(), m_rectLayer3.Height());
m_pdcLayer3->SelectObject(m_pdcLayer3->GetCurrentBitmap());
}
BOOL CMainFrame::OnCreateClient(LPCREATESTRUCT lpcs, CCreateContext* pContext)
{
// 创建三个视图,并将它们分别关联到三个CDC指针上
CRect rect;
GetClientRect(&rect);
BOOL bResult = FALSE;
bResult = m_wndSplitter.CreateStatic(this, 1, 3);
if (bResult)
{
bResult = m_wndSplitter.CreateView(0, 0, RUNTIME_CLASS(CLayer1View), CSize(m_rectLayer1.Width(), m_rectLayer1.Height()), pContext);
CLayer1View* pView1 = (CLayer1View*)m_wndSplitter.GetPane(0, 0);
pView1->SetDC(m_pdcLayer1, m_rectLayer1);
bResult = m_wndSplitter.CreateView(0, 1, RUNTIME_CLASS(CLayer2View), CSize(m_rectLayer2.Width(), m_rectLayer2.Height()), pContext);
CLayer2View* pView2 = (CLayer2View*)m_wndSplitter.GetPane(0, 1);
pView2->SetDC(m_pdcLayer2, m_rectLayer2);
bResult = m_wndSplitter.CreateView(0, 2, RUNTIME_CLASS(CLayer3View), CSize(m_rectLayer3.Width(), m_rectLayer3.Height()), pContext);
CLayer3View* pView3 = (CLayer3View*)m_wndSplitter.GetPane(0, 2);
pView3->SetDC(m_pdcLayer3, m_rectLayer3);
}
// 如果创建视图失败,则销毁CDC对象
if (!bResult)
{
delete m_pdcLayer1;
delete m_pdcLayer2;
delete m_pdcLayer3;
return FALSE;
}
return TRUE;
}
void CMainFrame::DrawLayers()
{
// 在三个CDC对象上分别绘制三个图层的内容
// We don't need to call BeginPaint or EndPaint here,
// because the CDCs hold their own contexts.
// Layer 1
m_pdcLayer1->FillSolidRect(m_rectLayer1, RGB(255, 255, 255));
m_pdcLayer1->TextOut(10, 10, _T("Layer 1"));
// Layer 2
m_pdcLayer2->FillSolidRect(m_rectLayer2, RGB(192, 192, 192));
m_pdcLayer2->Rectangle(10, 10, m_rectLayer2.Width() - 10, m_rectLayer2.Height() - 10);
m_pdcLayer2->TextOut(10, 10, _T("Layer 2"));
// Layer 3
m_pdcLayer3->FillSolidRect(m_rectLayer3, RGB(128, 128, 128));
m_pdcLayer3->Ellipse(10, 10, m_rectLayer3.Width() - 10, m_rectLayer3.Height() - 10);
m_pdcLayer3->TextOut(10, 10, _T("Layer 3"));
}
然后,分别创建三个视图类(CLayer1View、CLayer2View和CLayer3View),并在它们中间添加以下代码:
// CLayer1View/CLayer2View/CLayer3View.h
class CLayer1View : public CView
{
public:
CLayer1View() {}
DECLARE_DYNCREATE(CLayer1View)
// 设置CDC对象和绘制区域
void SetDC(CDC* pDC, CRect& rect) { m_pDC = pDC; m_rect = rect; }
// 重写
virtual void OnDraw(CDC* pDC) { pDC->BitBlt(0, 0, m_rect.Width(), m_rect.Height(), m_pDC, 0, 0, SRCCOPY); }
protected:
CDC* m_pDC;
CRect m_rect;
};
BEGIN_MESSAGE_MAP(CLayer1View, CView)
END_MESSAGE_MAP()
// CLayer1View/CLayer2View/CLayer3View.cpp
IMPLEMENT_DYNCREATE(CLayer1View, CView)
// 重写
void CLayer1View::OnDraw(CDC* pDC)
{
// 添加代码以分图层显示
pDC->BitBlt(0, 0, m_rect.Width(), m_rect.Height(), m_pDC, 0, 0, SRCCOPY);
}
接下来,在应用程序启动时,在主框架窗口类中的OnCreate函数中添加以下代码:
int CMyApp::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
if (CWinApp::OnCreate(lpCreateStruct) == -1)
return -1;
// 创建窗口并显示
m_pMainWnd = new CMainFrame;
if (!m_pMainWnd->Create(NULL, _T("MyApp"), WS_OVERLAPPEDWINDOW, CRect(0, 0, 800, 600)))
return FALSE;
m_pMainWnd->ShowWindow(SW_SHOWMAXIMIZED);
// 绘制三个图层
((CMainFrame*)m_pMainWnd)->DrawLayers();
return TRUE;
}
现在运行程序,即可看到如下所示的分图层绘图效果:
阅读全文
相关推荐
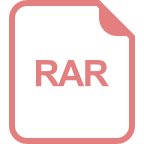
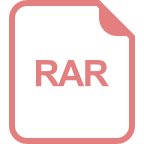
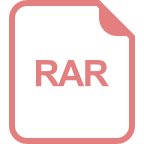
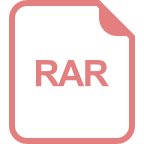
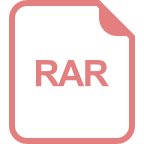
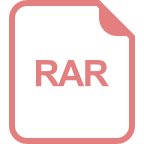
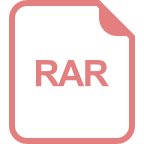
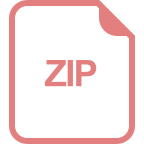
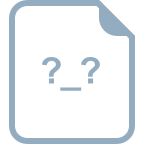
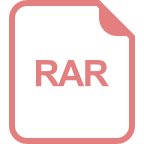
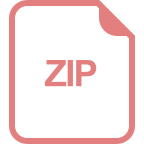
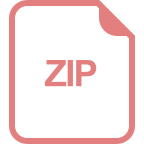
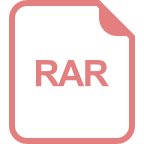
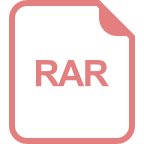
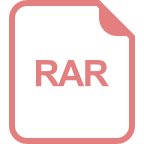
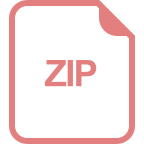
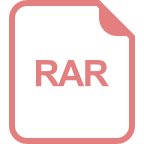