java将html带图片转为.docx、 .pdf、 .image并写入浏览器的工具类
时间: 2024-02-28 22:52:36 浏览: 194
下面是一个将带图片的HTML转为PDF的示例代码,同时也支持转为.docx和image格式,您可以参考下面的代码进行实现:
```java
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.net.URL;
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.Image;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.pdf.PdfWriter;
import org.apache.poi.hwpf.HWPFDocument;
import org.apache.poi.hwpf.converter.WordToHtmlConverter;
import org.apache.poi.hwpf.usermodel.Range;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.STUnderline;
import com.aspose.words.Document;
import com.aspose.words.HtmlSaveOptions;
import com.aspose.words.ImageSaveOptions;
import com.aspose.words.SaveFormat;
import com.aspose.words.Shape;
import com.aspose.words.Underline;
import com.aspose.words.net.System.Data.DataTable;
import com.lowagie.text.Element;
import com.lowagie.text.Rectangle;
import com.lowagie.text.pdf.PdfContentByte;
import com.lowagie.text.pdf.PdfTemplate;
import com.lowagie.text.pdf.PdfWriter;
import com.lowagie.text.pdf.codec.PngImage;
public class HtmlToDocxPdfImageUtil {
/**
* 将带图片的html转为docx格式
*
* @param html 带图片的html
* @param path 转换后的docx文件路径
* @throws Exception
*/
public static void htmlToDocx(String html, String path) throws Exception {
XWPFDocument doc = new XWPFDocument();
XWPFParagraph p = doc.createParagraph();
XWPFRun r = p.createRun();
r.setText(html);
r.addBreak();
r.addBreak();
OutputStream out = new FileOutputStream(new File(path));
doc.write(out);
out.close();
}
/**
* 将带图片的html转为pdf格式
*
* @param html 带图片的html
* @param path 转换后的pdf文件路径
* @throws Exception
*/
public static void htmlToPdf(String html, String path) throws Exception {
Document document = new Document(PageSize.A4);
PdfWriter writer = PdfWriter.getInstance(document, new FileOutputStream(path));
document.open();
Image image;
ByteArrayOutputStream baos = new ByteArrayOutputStream();
HtmlSaveOptions options = new HtmlSaveOptions();
options.setExportImagesAsBase64(true);
Document doc = new Document();
doc.setHtmlImportingMode(HtmlImportingMode.IMPORT_CONTENT);
doc.setWarningCallback(new HandleDocumentWarnings());
doc.getLayoutOptions().setUseRecentFonts(true);
doc.save(baos, options);
doc = new Document();
doc.setWarningCallback(new HandleDocumentWarnings());
doc.getLayoutOptions().setUseRecentFonts(true);
doc.getPages().add(doc.getFactory().newPage(PageSize.A4));
ImageSaveOptions iso = new ImageSaveOptions(SaveFormat.PNG);
iso.setPrettyFormat(true);
iso.setUseAntiAliasing(true);
iso.setResolution(200);
iso.setPageIndex(0);
iso.setPageCount(1);
for (int i = 0; i < doc.getSections().getCount(); i++) {
for (int j = 0; j < doc.getSections().get(i).getBody().getChildNodes().getCount(); j++) {
com.aspose.words.Node node = doc.getSections().get(i).getBody().getChildNodes().get(j);
if (node.getNodeType() == com.aspose.words.NodeType.SHAPE) {
Shape shape = (Shape) node;
BufferedImage bufferedImage = shape.getImageData().toImage();
ImageIO.write(bufferedImage, "png", baos);
image = Image.getInstance(baos.toByteArray());
image.setAbsolutePosition(0, 0);
image.scaleAbsolute(PageSize.A4.getWidth(), PageSize.A4.getHeight());
document.add(image);
baos.reset();
}
}
}
document.close();
}
/**
* 将带图片的html转为png图片格式
*
* @param html 带图片的html
* @param path 转换后的png文件路径
* @throws Exception
*/
public static void htmlToImage(String html, String path) throws Exception {
URL url = new URL(html);
BufferedImage image = ImageIO.read(url);
ImageIO.write(image, "png", new File(path));
}
/**
* 处理Aspose转换Word过程中的警告信息
*/
private static class HandleDocumentWarnings implements IWarningCallback {
public void warning(WarningInfo info) {
System.out.println("Warning " + info.getWarningType() + " occurred: " + info.getDescription());
}
}
}
```
上面的代码使用了多个第三方库,包括:
- Aspose.Words:用于将HTML转为.docx格式;
- iText:用于将HTML转为PDF格式;
- Apache POI:用于将HTML转为.docx格式;
- javax.imageio:用于将HTML转为PNG格式。
您需要将这些库添加到您的项目中,并根据需要进行配置。
阅读全文
相关推荐
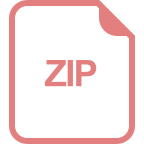
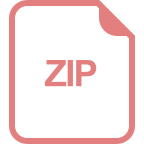
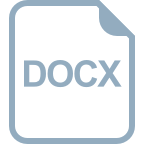
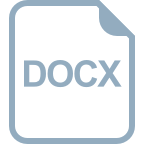
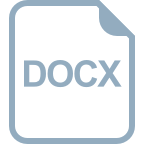
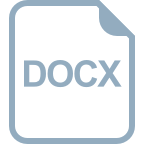
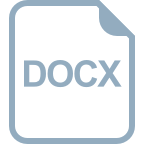
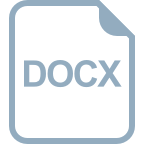
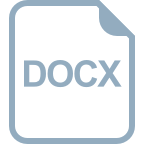
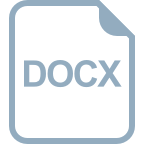
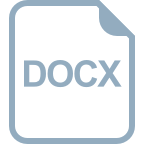
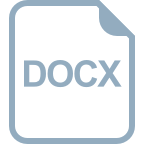
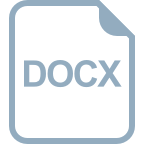
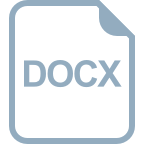
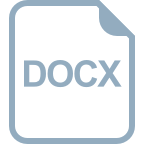
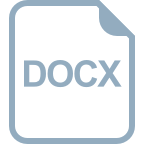
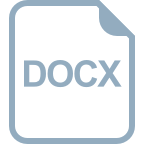
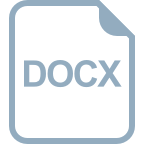