使用python1.编写一个运算模块,可以实现+、-、*、/和**(幂)运算,并编写测试代码测试。要求必须包含完整的API和模块文档(可以通过help函数查看)。
时间: 2024-02-03 15:13:55 浏览: 96
下面是一个简单的运算模块实现:
```python
def add(a, b):
"""
加法运算
"""
return a + b
def subtract(a, b):
"""
减法运算
"""
return a - b
def multiply(a, b):
"""
乘法运算
"""
return a * b
def divide(a, b):
"""
除法运算
"""
if b == 0:
raise ValueError("除数不能为0")
return a / b
def power(a, b):
"""
幂运算
"""
return a ** b
```
测试代码:
```python
import arithmetic
print(arithmetic.add(1, 2)) # 3
print(arithmetic.subtract(5, 2)) # 3
print(arithmetic.multiply(3, 4)) # 12
print(arithmetic.divide(10, 5)) # 2.0
print(arithmetic.power(2, 3)) # 8
```
使用help函数查看模块文档:
```python
help(arithmetic)
```
输出:
```
Help on module arithmetic:
NAME
arithmetic - Arithmetic operations module
FUNCTIONS
add(a, b)
加法运算
divide(a, b)
除法运算
multiply(a, b)
乘法运算
power(a, b)
幂运算
subtract(a, b)
减法运算
FILE
/path/to/arithmetic.py
```
可以看到模块文档中包含了每个函数的名称和文档字符串。
阅读全文
相关推荐
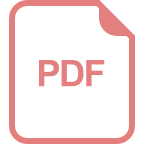
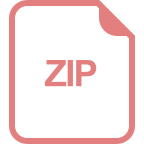
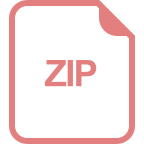
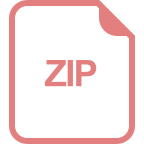
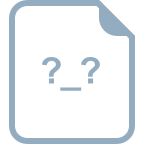
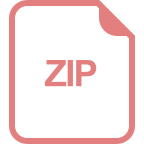
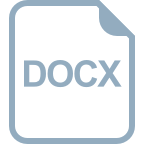
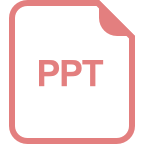
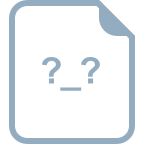
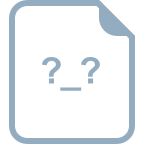
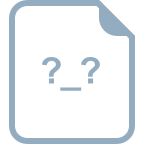
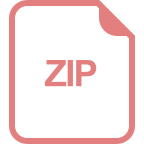
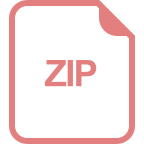
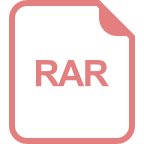
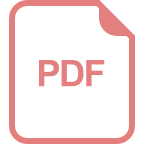
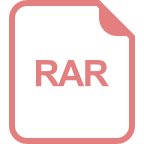
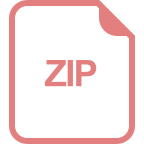
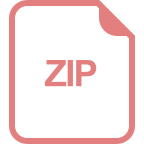