分享几个网页的源码和图片素材,要代码不要链接
时间: 2024-02-16 20:03:06 浏览: 196
好的,下面我分享几个网页的源码和图片素材,希望对你有所帮助:
1. 一个简单的个人主页
```html
<!DOCTYPE html>
<html>
<head>
<title>个人主页</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
<body>
<header>
<h1>My Personal Page</h1>
<nav>
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Blog</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
</header>
<main>
<section>
<h2>About Me</h2>
<p>Hi, I'm John. Lorem ipsum dolor sit amet, consectetur adipiscing elit. Duis eget justo ac tortor aliquet bibendum. Etiam nec viverra turpis. Nulla euismod sit amet eros id malesuada. Suspendisse auctor velit vel ex sagittis, at scelerisque metus tristique. Aliquam erat volutpat. Sed volutpat metus vel egestas faucibus. Donec sodales placerat turpis nec faucibus. Fusce vehicula, tellus vel luctus ornare, arcu eros molestie dui, eu elementum tellus dolor vitae velit. Nullam in enim dignissim, bibendum nisi ac, mattis lacus. Praesent vel mauris orci. Donec pretium neque mi, sit amet feugiat odio luctus vitae. Nam quis enim auctor, consectetur quam vel, condimentum purus. Praesent suscipit tellus sit amet nibh feugiat, sit amet imperdiet mi faucibus. Nullam malesuada, ipsum nec facilisis mollis, urna dui luctus ex, et lacinia libero odio sit amet nulla.</p>
</section>
<section>
<h2>Recent Blog Posts</h2>
<ul>
<li><a href="#">Post title 1</a></li>
<li><a href="#">Post title 2</a></li>
<li><a href="#">Post title 3</a></li>
</ul>
</section>
<section>
<h2>Contact Me</h2>
<form>
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<label for="message">Message:</label>
<textarea id="message" name="message" required></textarea>
<button type="submit">Send</button>
</form>
</section>
</main>
<footer>
<p>© 2022 John Doe</p>
</footer>
</body>
</html>
```
```css
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
header {
background-color: #333;
color: #fff;
padding: 20px;
}
header h1 {
margin: 0;
}
nav ul {
list-style: none;
margin: 0;
padding: 0;
display: flex;
justify-content: flex-end;
}
nav ul li {
margin: 0 10px;
}
nav ul li a {
color: #fff;
text-decoration: none;
}
main {
padding: 20px;
}
section {
margin-bottom: 20px;
}
section h2 {
margin-top: 0;
}
form {
display: flex;
flex-direction: column;
}
form label {
margin-bottom: 5px;
}
form input,
form textarea {
padding: 5px;
margin-bottom: 10px;
border-radius: 5px;
border: 1px solid #ccc;
}
form button[type="submit"] {
padding: 5px 10px;
background-color: #333;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
}
```
2. 一个简单的产品展示页面
```html
<!DOCTYPE html>
<html>
<head>
<title>Product Page</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
<body>
<header>
<h1>Product Name</h1>
<nav>
<ul>
<li><a href="#">Features</a></li>
<li><a href="#">Pricing</a></li>
<li><a href="#">Testimonials</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
</header>
<main>
<section>
<h2>Features</h2>
<ul>
<li>Lorem ipsum dolor sit amet</li>
<li>Consectetur adipiscing elit</li>
<li>Duis eget justo ac tortor aliquet bibendum</li>
<li>Etiam nec viverra turpis</li>
<li>Nulla euismod sit amet eros id malesuada</li>
<li>Suspendisse auctor velit vel ex sagittis</li>
<li>Aliquam erat volutpat</li>
<li>Sed volutpat metus vel egestas faucibus</li>
</ul>
</section>
<section>
<h2>Pricing</h2>
<ul>
<li>Basic Plan: $9.99/month</li>
<li>Pro Plan: $19.99/month</li>
<li>Enterprise Plan: $49.99/month</li>
</ul>
</section>
<section>
<h2>Testimonials</h2>
<ul>
<li>"This product is amazing! I can't believe how easy it is to use."</li>
<li>"I've tried other products, but this one is by far the best."</li>
<li>"I highly recommend this to anyone looking for a great product."</li>
</ul>
</section>
<section class="contact">
<h2>Contact Us</h2>
<form>
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<label for="message">Message:</label>
<textarea id="message" name="message" required></textarea>
<button type="submit">Send</button>
</form>
</section>
</main>
<footer>
<p>© 2022 Product Name</p>
</footer>
</body>
</html>
```
```css
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
header {
background-color: #333;
color: #fff;
padding: 20px;
}
header h1 {
margin: 0;
}
nav ul {
list-style: none;
margin: 0;
padding: 0;
display: flex;
justify-content: flex-end;
}
nav ul li {
margin: 0 10px;
}
nav ul li a {
color: #fff;
text-decoration: none;
}
main {
padding: 20px;
}
section {
margin-bottom: 20px;
}
section h2 {
margin-top: 0;
}
form {
display: flex;
flex-direction: column;
}
form label {
margin-bottom: 5px;
}
form input,
form textarea {
padding: 5px;
margin-bottom: 10px;
border-radius: 5px;
border: 1px solid #ccc;
}
form button[type="submit"] {
padding: 5px 10px;
background-color: #333;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
}
.contact {
background-color: #f2f2f2;
padding: 20px;
}
```
3. 一个简单的图片展示页面
```html
<!DOCTYPE html>
<html>
<head>
<title>Gallery Page</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
<body>
<header>
<h1>Gallery</h1>
<nav>
<ul>
<li><a href="#">Nature</a></li>
<li><a href="#">Animals</a></li>
<li><a href="#">City</a></li>
<li><a href="#">People</a></li>
</ul>
</nav>
</header>
<main>
<section>
<h2>Nature</h2>
<div class="gallery">
<img src="img/nature1.jpg" alt="Nature 1">
<img src="img/nature2.jpg" alt="Nature 2">
<img src="img/nature3.jpg" alt="Nature 3">
<img src="img/nature4.jpg" alt="Nature 4">
</div>
</section>
<section>
<h2>Animals</h2>
<div class="gallery">
<img src="img/animals1.jpg" alt="Animals 1">
<img src="img/animals2.jpg" alt="Animals 2">
<img src="img/animals3.jpg" alt="Animals 3">
<img src="img/animals4.jpg" alt="Animals 4">
</div>
</section>
<section>
<h2>City</h2>
<div class="gallery">
<img src="img/city1.jpg" alt="City 1">
<img src="img/city2.jpg" alt="City 2">
<img src="img/city3.jpg" alt="City 3">
<img src="img/city4.jpg" alt="City 4">
</div>
</section>
<section>
<h2>People</h2>
<div class="gallery">
<img src="img/people1.jpg" alt="People 1">
<img src="img/people2.jpg" alt="People 2">
<img src="img/people3.jpg" alt="People 3">
<img src="img/people4.jpg" alt="People 4">
</div>
</section>
</main>
<footer>
<p>© 2022 Gallery</p>
</footer>
</body>
</html>
```
```css
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
header {
background-color: #333;
color: #fff;
padding: 20px;
}
header h1 {
margin: 0;
}
nav ul {
list-style: none;
margin: 0;
padding: 0;
display: flex;
justify-content: flex-end;
}
nav ul li {
margin: 0 10px;
}
nav ul li a {
color: #fff;
text-decoration: none;
}
main {
padding: 20px;
}
section {
margin-bottom: 20px;
}
section h2 {
margin-top: 0;
}
.gallery {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(200px, 1fr));
grid-gap: 10px;
}
.gallery img {
width: 100%;
height: auto;
}
```
希望这些源码和图片素材能够对你有所帮助!
阅读全文
相关推荐
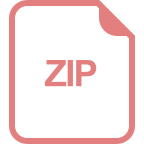

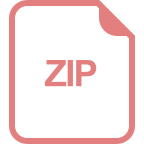
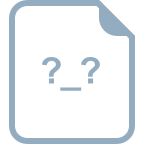
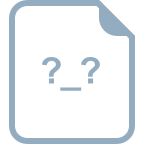
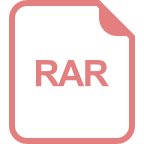
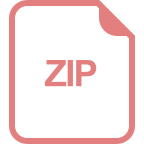
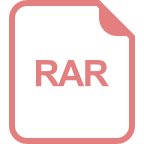
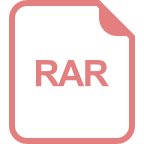
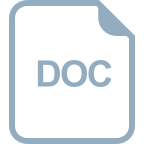
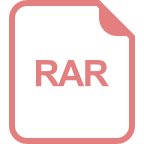
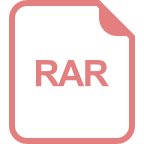
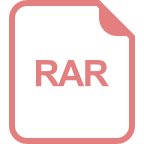
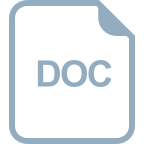
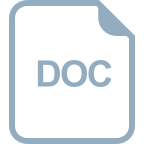
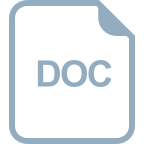
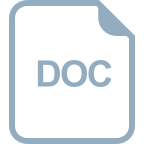