pycharm爬取知乎网页
时间: 2023-06-17 13:04:56 浏览: 96
要在PyCharm中爬取知乎网页,你可以按照以下步骤进行操作:
1. 安装必要的库
在PyCharm中打开终端,并使用pip安装以下库:
```
pip install requests
pip install beautifulsoup4
```
2. 编写爬虫代码
在PyCharm中创建一个新的Python文件,并使用以下代码作为爬虫代码的基础:
```
import requests
from bs4 import BeautifulSoup
url = 'https://www.zhihu.com/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.prettify())
```
3. 运行代码
在PyCharm中运行代码,并查看输出结果。
4. 解析网页内容
你可以使用BeautifulSoup库解析网页内容。例如,以下代码可以找到页面中的所有链接:
```
links = soup.find_all('a')
for link in links:
print(link.get('href'))
```
5. 更进一步
你可以使用Selenium库模拟用户行为,或使用Scrapy框架构建更为复杂的爬虫。不过,在进行任何爬虫活动之前,请确保遵守网站的使用条款和法律法规。
相关问题
pycharm爬取静态网页
可以使用PyCharm进行静态网页的爬取。以下是一个简单的示例代码,使用requests库来获取网页内容:
```python
import requests
url = 'https://example.com' # 替换为你要爬取的网页URL
# 发起GET请求并获取响应
response = requests.get(url)
# 打印网页内容
print(response.text)
```
你可以在PyCharm中创建一个新的Python文件,将以上代码复制粘贴到文件中,并替换`url`变量为你要爬取的网页URL。运行程序后,你将会看到网页的内容输出到控制台。
如果你需要解析网页内容,可以使用Python的HTML解析库,例如BeautifulSoup或lxml。
pycharm爬取网页文件
你可以使用 PyCharm 来编写爬取网页文件的代码。以下是一个简单的示例,演示如何使用 Python 的 requests 模块来获取网页内容并保存为文件:
```python
import requests
# 定义目标网页的 URL
url = "http://example.com"
# 发送 HTTP GET 请求并获取响应
response = requests.get(url)
# 检查响应状态码
if response.status_code == 200:
# 将网页内容保存为文件
with open("page.html", "wb") as file:
file.write(response.content)
print("网页文件保存成功!")
else:
print("无法访问网页!")
```
在这个示例中,我们使用了 requests 库来发送 HTTP GET 请求,并且将响应内容保存为名为 "page.html" 的文件。你可以根据自己的需求修改代码,并使用 PyCharm 运行该脚本来实现爬取网页文件的功能。
相关推荐
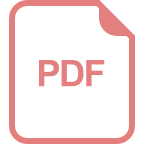












