在一个实验室预约管理系统中,用户登录后,可以查看自己的所有预约信息(包括序号,实验室名称,时间,状态(已签到或者爽约)等信息),可以进行实验室预约功能(要求不能在已经预约的时间段再次预约)。 代码要求: 1、
时间: 2024-11-05 07:24:27 浏览: 23
在一个实验室预约管理系统中,我们可以创建一个简单的类结构来模拟这个功能。首先,我们定义一个`LabSlot`类,表示一个实验室的预约时段:
```java
public class LabSlot {
private int slotId;
private String labName;
private Date startTime;
private Date endTime;
private boolean isReserved;
// 构造函数,getter和setter方法省略
}
```
然后,我们需要一个`User`类,它有一个关联的`List<LabSlot>`来存储用户的预约:
```java
import java.util.List;
public class User {
private String userId;
private List<LabSlot> appointments;
public User(String userId) {
this.userId = userId;
this.appointments = new ArrayList<>();
}
// 添加预约,查看预约信息等方法
public void addAppointment(LabSlot slot) {
if (slot.getIsReserved()) {
throw new IllegalArgumentException("Cannot reserve a slot that's already taken.");
}
// 检查时间冲突
for (LabSlot existing : appointments) {
if (existing.isOverlap(slot)) {
throw new IllegalStateException("Cannot reserve overlapping slots.");
}
}
appointments.add(slot);
}
// 其他getter和setter方法,以及查看所有预约的方法省略
}
// 辅助方法检查时间段是否冲突
private boolean isOverlap(LabSlot other) {
return !other.getStartTime().after(endTime) && !other.getEndTime().before(startTime);
}
```
在这个设计中,用户通过`User`类的实例操作自己的预约,并且系统会在添加新预约时自动检查冲突。为了实现登录后的功能,你需要一个`LoginManager`类来管理用户身份验证,然后将`User`实例与实际的登录状态关联起来。
阅读全文
相关推荐














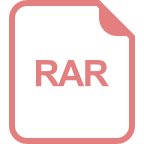



