qt 通过socket读取客户端发过来的信息,代码示例
时间: 2023-12-15 16:03:52 浏览: 17
好的,以下是一个简单的代码示例:
```c++
#include <QtNetwork>
int main()
{
QTcpSocket socket;
socket.connectToHost("127.0.0.1", 1234);
if (socket.waitForConnected()) {
qDebug() << "Connected!";
QByteArray data;
data.append("Hello server!");
socket.write(data);
socket.waitForBytesWritten();
socket.waitForReadyRead();
QByteArray response = socket.readAll();
qDebug() << "Response: " << response;
} else {
qWarning() << "Connection failed!";
}
return 0;
}
```
这个示例程序会连接到本地的 IP 地址为 127.0.0.1,端口号为 1234 的服务器。它会发送一条消息 "Hello server!",然后等待服务器的响应,并将收到的消息打印出来。当然,你可以根据自己的实际情况来修改这个代码示例。
相关问题
qt TCP Socket 客户端
下面是一个简单的 Qt TCP Socket 客户端示例代码:
```cpp
#include <QTcpSocket>
#include <QHostAddress>
void MyClient::connectToHost(const QString& ip, int port)
{
m_socket = new QTcpSocket(this);
connect(m_socket, &QTcpSocket::connected, this, &MyClient::onConnected);
connect(m_socket, &QTcpSocket::disconnected, this, &MyClient::onDisconnected);
connect(m_socket, &QTcpSocket::readyRead, this, &MyClient::onReadyRead);
m_socket->connectToHost(QHostAddress(ip), port);
}
void MyClient::sendData(const QByteArray& data)
{
m_socket->write(data);
}
void MyClient::onConnected()
{
qDebug() << "Connected to server";
}
void MyClient::onDisconnected()
{
qDebug() << "Disconnected from server";
}
void MyClient::onReadyRead()
{
QByteArray data = m_socket->readAll();
qDebug() << "Received data: " << data;
}
```
在上面的代码中,我们首先创建了一个 QTcpSocket 对象,并连接了它的一些信号和槽。然后,我们通过调用 `connectToHost` 函数连接到服务器。一旦连接建立,`onConnected` 槽将被调用。然后,我们可以通过调用 `sendData` 函数向服务器发送数据。当服务器发送数据时,`onReadyRead` 槽将被调用,我们可以在其中读取数据。最后,当连接断开时,`onDisconnected` 槽将被调用。
qt实现客户端和服务端相互发送接收信息的代码
以下是一个简单的 Qt 客户端和服务端实现互相发送接收信息的示例代码:
服务端代码:
```cpp
#include <QTcpServer>
#include <QTcpSocket>
int main(int argc, char *argv[])
{
QTcpServer server;
server.listen(QHostAddress::Any, 12345); // 监听所有可用的网络接口上的 12345 端口
QObject::connect(&server, &QTcpServer::newConnection, [&]()
{
QTcpSocket *socket = server.nextPendingConnection(); // 获取新连接的套接字
QObject::connect(socket, &QTcpSocket::readyRead, [&]()
{
QByteArray recvData = socket->readAll(); // 读取接收到的数据
qDebug() << "Recv:" << recvData;
socket->write(recvData); // 回复接收到的数据
});
});
return app.exec();
}
```
客户端代码:
```cpp
#include <QTcpSocket>
int main(int argc, char *argv[])
{
QTcpSocket socket;
socket.connectToHost("127.0.0.1", 12345); // 连接到 localhost 上的 12345 端口
QObject::connect(&socket, &QTcpSocket::connected, [&]()
{
QByteArray sendData = "Hello, server!"; // 要发送的数据
socket.write(sendData); // 发送数据
});
QObject::connect(&socket, &QTcpSocket::readyRead, [&]()
{
QByteArray recvData = socket.readAll(); // 读取接收到的数据
qDebug() << "Recv:" << recvData;
});
return app.exec();
}
```
在运行上述代码之前,需要在项目的 .pro 文件中添加以下代码:
```pro
QT += network
```
这样才能使用 Qt 的网络模块。
相关推荐
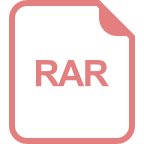












