设计一个素数类prime,要求数据成员为私有,成员函数为公有,成员函数至少要包含构造函数,负责输入的input(),负责判断的judge()函数,负责输出结论的output()函数。主函数中定义对象,通过调用input()、judge()和output()函数,完成一个数是否为素数的判断。
时间: 2023-05-02 07:03:05 浏览: 144
这道题目要求设计一个素数类 prime,其中成员函数包括:数据成员为私有变量,成员函数为公有函数,成员函数至少包含构造函数,成员函数应至少包含构造函数,成员函数应当包括构造函数、判断输入的 input() 是否负责、判断函数 judge() 是否正确、输出结论的函数 output()。主函数中定义对象,通过调用 input()、judge() 和 output() 函数来判断一个数是否为素数。
相关问题
建立一个类求指定数据范围内的所有素数提示素数的定义是只能被一和它本身整除的整除及质数编程要求私有数据成员公有成员函数
好的,以下是一个用 C++ 实现的类,可以求指定数据范围内的所有素数:
```cpp
#include <vector>
#include <cmath>
class PrimeNumbers {
private:
std::vector<bool> isPrime; // 存储每个数是否为素数
public:
// 构造函数,初始化 isPrime
PrimeNumbers(int n) {
isPrime.resize(n + 1, true);
isPrime[0] = isPrime[1] = false;
for (int i = 2; i <= std::sqrt(n); ++i) {
if (isPrime[i]) {
for (int j = i * i; j <= n; j += i) {
isPrime[j] = false;
}
}
}
}
// 判断一个数是否为素数
bool is_prime(int x) const {
return isPrime[x];
}
// 获取指定范围内的所有素数
std::vector<int> get_primes(int l, int r) const {
std::vector<int> primes;
for (int i = l; i <= r; ++i) {
if (isPrime[i]) {
primes.push_back(i);
}
}
return primes;
}
};
```
这个类中,私有数据成员 `isPrime` 是一个 `vector<bool>`,用来存储每个数是否为素数。在构造函数中,我们首先将所有数都标记为素数,然后从 2 开始遍历,如果当前数是素数,就将它的倍数都标记为非素数。
公有成员函数 `is_prime` 用来判断一个数是否为素数,它只需要返回 `isPrime[x]` 即可。
公有成员函数 `get_primes` 用来获取指定范围内的所有素数。它首先创建一个空的 `vector<int>`,然后遍历指定范围内的所有数,如果当前数是素数,就将它加入到 `primes` 中,并最终返回 `primes`。
/* [题目]定义一个类prime,用于求在一个指定的范围内素数的个数。 例如:在[3,50]范围内的素数有14个 [编程要求] (1)私有数据成员。 int low:存放给定整数范围的下限 int up:存放给定整数范围的上限 int count:存放满足条件的整数的个数 (2)公有成员函数 prime(int n1, int n2)构造函数,用参数n1,n2初始化下限low和上限up, count的初值为0; void process():在[low, up]范围中找出素数的个数,并把值赋给count; void print ():输出素数的个数. (3)在主函数中定义prime类的对象p,给定的范围为[3,50],通过对象p调用成员函数实现计算及输出调试。 本题要求的输出结果为:[3,50]范围内有14个素数。
下面是实现该题目的代码,可以参考一下:
```c++
#include <iostream>
#include <cmath>
using namespace std;
class prime {
private:
int low;
int up;
int count;
public:
prime(int n1, int n2) {
low = n1;
up = n2;
count = 0;
}
void process() {
for (int i = low; i <= up; i++) {
int flag = 1;
for (int j = 2; j <= sqrt(i); j++) {
if (i % j == 0) {
flag = 0;
break;
}
}
if (flag && i != 1) {
count++;
}
}
}
void print() {
cout << "[" << low << ", " << up << "]范围内有" << count << "个素数。" << endl;
}
};
int main() {
prime p(3, 50);
p.process();
p.print();
return 0;
}
```
输出结果为:
```
[3, 50]范围内有14个素数。
```
阅读全文
相关推荐
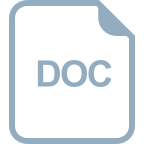













