使用python写一个程序,实现快捷键识别选定区域内的文字并转入剪贴板
时间: 2024-05-03 16:22:08 浏览: 13
以下是一个使用Python和PyQt5库实现快捷键识别选定区域内的文字并转入剪贴板的示例程序:
```python
import sys
import pyautogui
import keyboard
from PyQt5.QtWidgets import QApplication, QWidget
from PyQt5.QtCore import Qt, QTimer
class TextRecognition(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setGeometry(300, 300, 300, 100)
self.setWindowTitle('Text Recognition')
self.setWindowFlags(Qt.WindowStaysOnTopHint)
self.timer = QTimer(self)
self.timer.timeout.connect(self.recognize_text)
self.show()
def recognize_text(self):
x, y, width, height = pyautogui.locateOnScreen('selected_area.png')
if x is not None:
pyautogui.click(x + 5, y + 5)
pyautogui.hotkey('ctrl', 'c')
self.timer.stop()
self.close()
def keyPressEvent(self, event):
if event.key() == Qt.Key_F1:
self.timer.start(100)
app = QApplication(sys.argv)
text_recognition = TextRecognition()
sys.exit(app.exec_())
```
在这个程序中,我们使用了`pyautogui`库来定位选定区域的位置并模拟鼠标点击,使用`keyboard`库来监听F1键的按下事件,使用`QTimer`来定时执行识别文本的任务。
当用户按下F1键时,程序会启动一个定时器,每100毫秒执行一次`recognize_text`方法。在这个方法中,我们使用`pyautogui.locateOnScreen`函数来查找屏幕上是否存在名为`selected_area.png`的图片,如果存在,则执行鼠标点击和复制操作,并停止定时器和关闭程序。
注意,为了让程序始终保持在最顶层,我们使用了`setWindowFlags(Qt.WindowStaysOnTopHint)`方法来设置窗口的标志位。此外,为了让程序接收到键盘事件,我们重写了`keyPressEvent`方法,并在其中判断是否按下了F1键。
相关推荐
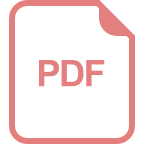
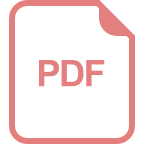














