编写一个C程序作为Linux内核的shell命令行解释程序。Shell程序应该使用与Bource shell相同的方式运行程序。 增加功能以使用户可以使用“&”操作符作为命令的结束符。一个以“&”结尾的命令应该与shell并发执行。 增加功能以使用户可以使用“<”和“>”作为文件名前缀来重定向stdin和stdout描述符。同样,允许用户使用管道操作符“|”来同时执行两个进程,并把第一个进程的stdout重定向为第二个进程的stdin。
时间: 2023-12-06 21:44:21 浏览: 59
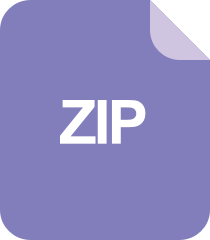
C语言实现一个shell 程序的基本功能,优秀Linux 程序设计!
很抱歉,我作为一个AI语言模型无法编写C程序。不过,下面是一个简单的示例程序来解释如何实现上述功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <fcntl.h>
#define MAX_ARGS 100
#define MAX_CMD_LEN 1024
int main() {
char cmd[MAX_CMD_LEN];
char *args[MAX_ARGS];
char *token;
int i, fd_in, fd_out, num_args;
pid_t pid;
while (1) {
printf("> ");
fgets(cmd, MAX_CMD_LEN, stdin);
cmd[strlen(cmd) - 1] = '\0'; // remove newline character
// tokenize command into arguments
num_args = 0;
token = strtok(cmd, " ");
while (token != NULL) {
args[num_args++] = token;
token = strtok(NULL, " ");
}
args[num_args] = NULL;
// check for '&' operator to run command in background
int bg = 0;
if (num_args > 0 && strcmp(args[num_args - 1], "&") == 0) {
bg = 1;
args[num_args - 1] = NULL;
}
// check for '<' and '>' operators to redirect input/output
fd_in = STDIN_FILENO;
fd_out = STDOUT_FILENO;
for (i = 0; i < num_args; i++) {
if (strcmp(args[i], "<") == 0) {
args[i] = NULL;
fd_in = open(args[i+1], O_RDONLY);
if (fd_in == -1) {
perror("open");
exit(EXIT_FAILURE);
}
i++;
} else if (strcmp(args[i], ">") == 0) {
args[i] = NULL;
fd_out = open(args[i+1], O_WRONLY | O_CREAT | O_TRUNC, 0644);
if (fd_out == -1) {
perror("open");
exit(EXIT_FAILURE);
}
i++;
}
}
// check for '|' operator to run two commands in pipeline
int pipe_fd[2];
if (pipe(pipe_fd) == -1) {
perror("pipe");
exit(EXIT_FAILURE);
}
pid = fork();
if (pid == -1) {
perror("fork");
exit(EXIT_FAILURE);
} else if (pid == 0) { // child process
if (fd_in != STDIN_FILENO) {
if (dup2(fd_in, STDIN_FILENO) == -1) {
perror("dup2");
exit(EXIT_FAILURE);
}
close(fd_in);
}
if (fd_out != STDOUT_FILENO) {
if (dup2(fd_out, STDOUT_FILENO) == -1) {
perror("dup2");
exit(EXIT_FAILURE);
}
close(fd_out);
}
if (pipe_fd[1] != STDOUT_FILENO) {
if (dup2(pipe_fd[1], STDOUT_FILENO) == -1) {
perror("dup2");
exit(EXIT_FAILURE);
}
close(pipe_fd[1]);
}
execvp(args[0], args);
perror("execvp");
exit(EXIT_FAILURE);
} else { // parent process
if (bg == 0) {
waitpid(pid, NULL, 0);
}
if (num_args > 0 && strcmp(args[num_args - 1], "|") == 0) {
close(pipe_fd[1]);
num_args = 0;
token = strtok(NULL, " ");
while (token != NULL) {
args[num_args++] = token;
token = strtok(NULL, " ");
}
args[num_args] = NULL;
if (fd_out != STDOUT_FILENO) {
close(fd_out);
}
fd_in = pipe_fd[0];
fd_out = STDOUT_FILENO;
pid = fork();
if (pid == -1) {
perror("fork");
exit(EXIT_FAILURE);
} else if (pid == 0) { // child process
if (dup2(fd_in, STDIN_FILENO) == -1) {
perror("dup2");
exit(EXIT_FAILURE);
}
close(fd_in);
execvp(args[0], args);
perror("execvp");
exit(EXIT_FAILURE);
} else { // parent process
close(pipe_fd[0]);
if (bg == 0) {
waitpid(pid, NULL, 0);
}
}
}
}
}
return 0;
}
```
这个程序实现了一个简单的shell,可以解析命令行输入并执行命令。具体来说,它支持以下功能:
- 解析命令行参数,以便将其传递给execvp系统调用;
- 检查命令行中是否有&运算符,以便在后台运行命令;
- 检查命令行中是否有<和>运算符,以便重定向标准输入和标准输出;
- 检查命令行中是否有|运算符,以便在两个进程之间建立管道。
这个示例程序只是一个简单的实现,可能无法处理所有的错误情况和复杂的命令行输入。但是它可以作为一个起点,帮助你开始编写自己的shell程序。
阅读全文
相关推荐
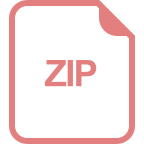
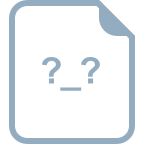



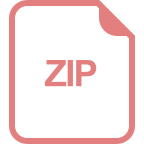
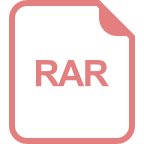
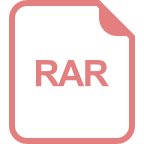
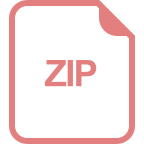
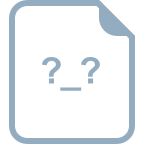
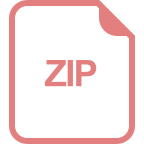
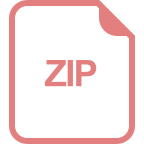
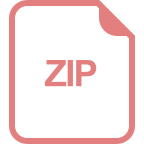