java使用webservice的demo
时间: 2023-09-18 18:14:28 浏览: 119
这是一个简单的Java使用WebService的示例:
1. 创建一个Java项目并在项目中添加以下依赖:
```xml
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.bind</groupId>
<artifactId>jaxb-impl</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.bind</groupId>
<artifactId>jaxb-core</artifactId>
<version>2.3.0.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.bind</groupId>
<artifactId>jaxb-xjc</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.ws</groupId>
<artifactId>jaxws-rt</artifactId>
<version>2.3.1</version>
</dependency>
```
2. 创建一个Java类作为我们的WebService客户端:
```java
import javax.xml.namespace.QName;
import javax.xml.ws.Service;
import java.net.URL;
public class HelloWorldClient {
public static void main(String[] args) throws Exception {
URL url = new URL("http://localhost:8080/HelloWorld?wsdl");
QName qname = new QName("http://webservice.example.com/", "HelloWorldImplService");
Service service = Service.create(url, qname);
HelloWorld hello = service.getPort(HelloWorld.class);
System.out.println(hello.sayHello("World"));
}
}
```
3. 创建一个Java接口作为我们的WebService服务:
```java
import javax.jws.WebMethod;
import javax.jws.WebService;
@WebService
public interface HelloWorld {
@WebMethod
String sayHello(String name);
}
```
4. 创建一个Java类作为我们的WebService服务实现:
```java
import javax.jws.WebService;
@WebService(endpointInterface = "com.example.webservice.HelloWorld")
public class HelloWorldImpl implements HelloWorld {
public String sayHello(String name) {
return "Hello " + name + "!";
}
}
```
5. 在Eclipse中运行我们的WebService服务端
6. 在Eclipse中运行我们的WebService客户端
7. 我们应该能够在控制台看到以下输出:
```
Hello World!
```
这就是一个简单的Java使用WebService的示例。
阅读全文
相关推荐
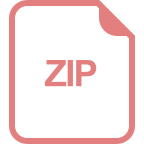
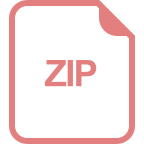
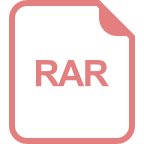
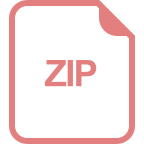
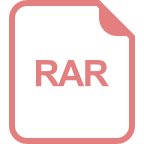
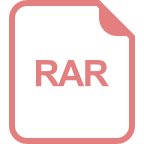
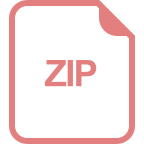



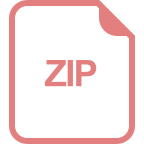