JSONObject json2 = JSONObject.parseObject(request.read()); 这行代码什么意思
时间: 2023-04-01 11:02:38 浏览: 61
这行代码的意思是将从请求中读取的字符串转换为 JSONObject 对象。其中,JSONObject 是一个 Java 类,parseObject() 是该类中的一个静态方法,用于将字符串转换为 JSONObject 对象。request.read() 是一个方法,用于从请求中读取字符串。
相关问题
DataBufferUtils.join(exchange.getRequest().getBody().defaultIfEmpty(factory.wrap(new EmptyByteBuf(factory.getByteBufAllocator())))) .flatMap(dataBuffer -> { byte[] bytes = new byte[dataBuffer.readableByteCount()]; dataBuffer.read(bytes); System.out.println(Arrays.toString(bytes)); String bodyString = new String(bytes, StandardCharsets.UTF_8); System.out.println(bodyString); JSONObject jsonObject = JSON.parseObject(bodyString); jsonObject.put(FieldUtils.USER_KEY, finalUserId); bodyString = jsonObject.toJSONString(); System.out.println(bodyString); System.out.println(Arrays.toString(bodyString.getBytes(StandardCharsets.UTF_8))); String finalBodyString = bodyString; exchange.getAttributes().put("POST_BODY", finalBodyString); DataBufferUtils.release(dataBuffer); Flux<DataBuffer> cachedFlux = Flux.defer(() -> { DataBuffer buffer = exchange.getResponse().bufferFactory().wrap(bytes); return Mono.just(buffer); }); ServerHttpRequest mutatedRequest = new ServerHttpRequestDecorator(exchange.getRequest()) { @Override public Flux<DataBuffer> getBody() { return cachedFlux; } }; return chain.filter(exchange.mutate().request(mutatedRequest).build()); });json转换有问题吗,怎么解决
这段代码中涉及到对请求体进行解析和转换成 JSON 对象,并且在修改后重新设置到请求体中。但是没有看到你提到的具体的问题,可以提供更详细的错误信息或者具体的问题描述吗?
另外,如果你使用的是 Spring WebFlux,也可以直接使用 `@RequestBody` 注解将请求体转换成对应的 Java 对象,避免手动解析和转换。像这样:
```java
@PostMapping("/api/user")
public Mono<User> createUser(@RequestBody User user) {
// 处理用户创建逻辑
}
```
Java后台Servlet如何获取请求体中为JSON格式的数据项
在Java后台Servlet中,我们可以通过以下方式获取请求体中为JSON格式的数据项:
```java
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
BufferedReader reader = request.getReader();
StringBuilder sb = new StringBuilder();
char[] buf = new char[1024];
int len;
while ((len = reader.read(buf)) != -1) {
sb.append(buf, 0, len);
}
String requestBody = sb.toString();
JSONObject jsonObject = JSONObject.parseObject(requestBody);
String dataItem = jsonObject.getString("dataItem");
// 处理数据项
}
```
在以上代码中,我们首先获取到请求体的输入流,然后通过BufferedReader逐行读取输入流中的数据。接着将读取到的数据拼接成一个完整的字符串,即为请求体的内容。然后我们将请求体数据解析成JSONObject对象,然后通过getString方法获取其中的数据项。
需要注意的是,在使用以上方式获取请求体数据时,需要保证请求头中的Content-Type为application/json,否则无法正确解析请求体数据。同时,需要确保请求体中的JSON数据项名称和Java对象属性名称一致,否则无法正确解析。
相关推荐
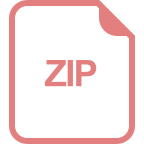











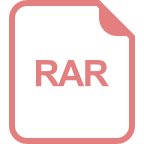