逐行解释代码MOV R0,#1;BL LOOP;MOV R0,#3;LOOP MOV R0,#2
时间: 2023-09-16 20:10:07 浏览: 354
这段代码是ARM汇编语言的代码,它的作用是将寄存器R0的值分别设置为1、2和3,然后跳转到LOOP标签处执行循环。
具体解释如下:
1. MOV R0,#1; 将立即数1存储到寄存器R0中;
2. BL LOOP; 跳转到LOOP标签处执行循环;
3. MOV R0,#3; 跳出循环后,将立即数3存储到寄存器R0中;
4. LOOP 标签,表示循环的开始;
5. MOV R0,#2; 在循环中,将立即数2存储到寄存器R0中;
6. 循环结束后,程序会跳转回LOOP标签处,再次执行循环。
相关问题
data segment x db '-' sign db 0 M db 0 data ends stack segment db 20 dup(?) stack ends code segment assume ds:data,cs:code,ss:stack start: mov ax,data mov ds,ax input: mov ah,1 int 21h mov bl,al cmp bl,x je change cmp bl,0dh jz deal1 mov cl,0 sub al,30h mov cl,al xor bl,bl mov bl,0ah sub al,al mov al,M mul bl mov M,0 add M,al add M,cl jmp input deal1: cmp sign,1 jz outx deal2: mov dl,M sub cx,cx mov cx,1 mov bl,10 print: mov ah,0 mov al,dl div bl push ax cmp al,0 jle endl mov dl,al inc cx jmp print endl: pop dx xchg dh,dl add dl,30h mov ah,2 int 21h loop endl jmp exit change: mov sign,1 jmp input outx: mov ah,2 mov dl,x int 21h jmp deal2 exit: mov ax,4c00h int 21h code ends end start是什么意思
这段代码是8086汇编语言编写的程序,其中各个段的含义如下:
- data segment: 存放数据的段,x、sign、M都是存储数据的变量。
- stack segment: 栈的段,存放程序运行过程中的临时变量。
- code segment: 存放程序代码的段,其中包含了主函数start。
其中end start表示程序的结束点,即程序结束后会跳转到操作系统的程序开始执行。
Use the following codes as the beginning of your program. Write a sort program to sort these ten numbers from largest to smallest, then save them to memory. For ARMSim#, the sorted number also need to be displayed in the console and LCD screen (use the Embest Board Plug‐In). MOV r0, #0x00002000 MOV r1, #18 STR r1, [r0],#4 MOV r1, #6 STR r1, [r0],#4 MOV r1, #2 STR r1, [r0],#4 MOV r1, #8 STR r1, [r0],#4 MOV r1, #16 STR r1, [r0],#4 MOV r1, #10 STR r1, [r0],#4 MOV r1, #14 STR r1, [r0],#4 MOV r1, #4 STR r1, [r0],#4 MOV r1, #20 STR r1, [r0],#4 MOV r1, #12 STR r1, [r0],#4
Sure, here's a sample program in ARM assembly language that sorts the 10 numbers in descending order using the bubble sort algorithm and saves them to memory starting from the address 0x00002000. The sorted numbers are also displayed in the console and LCD screen using the Embest Board Plug-In.
```
; Define constants
MEMORY_START EQU 0x00002000
NUM_ELEMENTS EQU 10
LCD_BASE_ADDRESS EQU 0x12345678 ; Replace with actual address
CONSOLE_BASE_ADDRESS EQU 0x87654321 ; Replace with actual address
; Define variables
array SPACE NUM_ELEMENTS * 4
temp SPACE 4
; Main program
MOV r0, #MEMORY_START ; r0 = starting address of array
LDR r1, =array ; r1 = address of array
LDR r2, =NUM_ELEMENTS ; r2 = number of elements in array
BL bubble_sort ; Sort the array
BL display_numbers ; Display the sorted numbers
exit:
B exit ; Infinite loop
; Bubble sort implementation
bubble_sort:
PUSH {r4, lr} ; Save registers
MOV r3, #1 ; Boolean value to indicate when a swap has occurred
outer_loop:
MOV r4, #0 ; Clear the swap flag
MOV r5, #1 ; r5 = index of first element
inner_loop:
CMP r5, r2 ; Compare r5 with number of elements
BGE outer_loop ; If r5 >= number of elements, exit inner loop
LDR r6, [r1, r5, LSL #2] ; r6 = array[r5]
LDR r7, [r1, r5-1, LSL #2] ; r7 = array[r5-1]
CMP r6, r7 ; Compare array[r5] with array[r5-1]
BGE no_swap ; If array[r5] >= array[r5-1], no need to swap
STR r6, [r1, r5-1, LSL #2] ; Swap array[r5] and array[r5-1]
STR r7, [r1, r5, LSL #2]
MOV r4, #1 ; Set the swap flag
no_swap:
ADD r5, r5, #1 ; Increment the index
B inner_loop ; Continue inner loop
CMP r4, #1 ; Check if a swap has occurred
BNE outer_loop ; If no swap, the array is sorted
POP {r4, pc} ; Restore registers and return
; Display the sorted numbers in console and LCD screen
display_numbers:
PUSH {r4, lr} ; Save registers
MOV r4, #0 ; r4 = index of first element
display_loop:
CMP r4, r2 ; Compare r4 with number of elements
BGE exit_display ; If r4 >= number of elements, exit display loop
LDR r5, [r1, r4, LSL #2] ; r5 = array[r4]
BL display_console ; Display the number in console
BL display_lcd ; Display the number in LCD
ADD r4, r4, #1 ; Increment the index
B display_loop ; Continue display loop
exit_display:
POP {r4, pc} ; Restore registers and return
; Display a number in console
display_console:
; Code to display the number in console using the CONSOLE_BASE_ADDRESS
; Replace with actual code
BX lr ; Return
; Display a number in LCD screen
display_lcd:
; Code to display the number in LCD using the LCD_BASE_ADDRESS
; Replace with actual code
BX lr ; Return
```
Please note that this is just a sample program, and it may not work as-is in your specific environment. You may need to adjust the memory addresses and display functions based on your hardware and software setup.
阅读全文
相关推荐
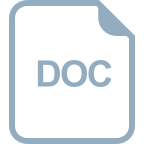
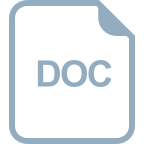
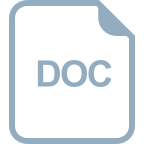














