通过springboot使用resttenple 调用第三方接口事例
时间: 2023-11-08 09:47:17 浏览: 42
好的,下面是一个使用 Spring Boot 和 RestTemplate 调用第三方接口的示例:
1. 首先在 pom.xml 文件中添加以下依赖:
```xml
<dependencies>
<!-- Spring Boot Web Starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Boot Test Starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- Spring Boot RestTemplate Starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web-services</artifactId>
</dependency>
</dependencies>
```
2. 编写一个 RestTemplate 配置类:
```java
@Configuration
public class RestTemplateConfig {
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
```
3. 编写一个使用 RestTemplate 调用第三方接口的服务类:
```java
@Service
public class ThirdPartyService {
private final RestTemplate restTemplate;
@Autowired
public ThirdPartyService(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
public String getDataFromThirdPartyApi() {
String apiUrl = "http://example.com/api/data";
ResponseEntity<String> responseEntity = restTemplate.getForEntity(apiUrl, String.class);
if (responseEntity.getStatusCode() == HttpStatus.OK) {
return responseEntity.getBody();
} else {
throw new RuntimeException("Something went wrong while calling third party API");
}
}
}
```
4. 最后,在 Controller 中注入 ThirdPartyService 并调用相关方法:
```java
@RestController
public class MyController {
private final ThirdPartyService thirdPartyService;
@Autowired
public MyController(ThirdPartyService thirdPartyService) {
this.thirdPartyService = thirdPartyService;
}
@GetMapping("/data")
public String getData() {
return thirdPartyService.getDataFromThirdPartyApi();
}
}
```
这样,在浏览器中访问 http://localhost:8080/data 就会调用第三方接口并返回数据了。
相关推荐
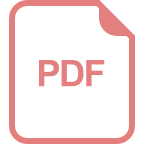














