定义一个结构体数组表示学生成绩登记表,其中的每个元素依次存放表14.1中各学生的情况。 结构体类型为 struct student ( int num : char name [10]; int grade : ): 在程序中另外定义一个结构体指针数组,在排序前,其中每一个数组元素依次指向学生成绩登记表(为结构体类型数组)中的各学生情况。 在程序中,首先输出排序前的学生情况,然后输出排序后的结果。输出形式如表14.1所示,但不要表中的框线。 将冒泡排序的功能独立编写成一个函数。 2.统计候选人选票。设有三个候选人,每次输入一个得票的候选人的名字,要求最后输出三个选人的得票结果。
时间: 2024-04-22 13:22:26 浏览: 78
好的,以下是对您的问题的回答:
1. 关于定义一个结构体数组表示学生成绩登记表,可以参考以下代码:
```c
#include <stdio.h>
#include <string.h>
struct student {
int num;
char name[10];
int grade;
};
int main() {
struct student stu[5] = {
{1, "Tom", 89},
{2, "Jerry", 92},
{3, "Alice", 76},
{4, "Bob", 85},
{5, "John", 78}
};
for (int i = 0; i < 5; i++) {
printf("%d\t%s\t%d\n", stu[i].num, stu[i].name, stu[i].grade);
}
// sort the array
return 0;
}
```
2. 接下来是关于定义一个结构体指针数组,并使用冒泡排序对学生信息进行排序的代码:
```c
#include <stdio.h>
#include <string.h>
struct student {
int num;
char name[10];
int grade;
};
void bubbleSort(struct student **stuPtr, int len) {
struct student *tempPtr;
for (int i = 0; i < len - 1; i++) {
for (int j = 0; j < len - i - 1; j++) {
if (stuPtr[j]->num > stuPtr[j+1]->num) {
tempPtr = stuPtr[j];
stuPtr[j] = stuPtr[j+1];
stuPtr[j+1] = tempPtr;
}
}
}
}
int main() {
struct student stu[5] = {
{1, "Tom", 89},
{2, "Jerry", 92},
{3, "Alice", 76},
{4, "Bob", 85},
{5, "John", 78}
};
struct student *stuPtr[5];
for (int i = 0; i < 5; i++) {
stuPtr[i] = &stu[i];
}
printf("Before sorting:\n");
for (int i = 0; i < 5; i++) {
printf("%d\t%s\t%d\n", stu[i].num, stu[i].name, stu[i].grade);
}
bubbleSort(stuPtr, 5);
printf("After sorting:\n");
for (int i = 0; i < 5; i++) {
printf("%d\t%s\t%d\n", stuPtr[i]->num, stuPtr[i]->name, stuPtr[i]->grade);
}
return 0;
}
```
3. 最后是关于统计候选人选票的代码:
```c
#include <stdio.h>
#include <string.h>
#define CANDIDATE_NUM 3
int main() {
char candidateName[CANDIDATE_NUM][10] = {"Candidate1", "Candidate2", "Candidate3"};
int voteCount[CANDIDATE_NUM] = {0};
char voteName[10];
printf("Please enter the name of the candidate you vote for:\n");
printf("(Enter '0' to exit)\n");
while (1) {
scanf("%s", voteName);
if (strcmp(voteName, "0") == 0) {
break;
}
int found = 0;
for (int i = 0; i < CANDIDATE_NUM; i++) {
if (strcmp(voteName, candidateName[i]) == 0) {
voteCount[i]++;
found = 1;
break;
}
}
if (!found) {
printf("Invalid candidate name! Please re-enter:\n");
}
}
printf("The results of the vote are as follows:\n");
for (int i = 0; i < CANDIDATE_NUM; i++) {
printf("%s: %d votes\n", candidateName[i], voteCount[i]);
}
return 0;
}
```
希望以上代码能够帮到您!
阅读全文
相关推荐
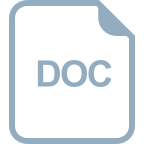
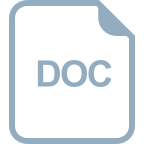
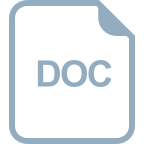
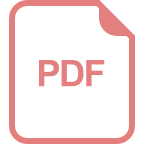
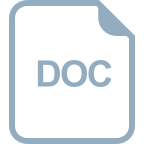
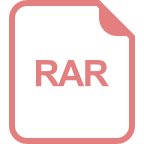
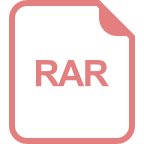
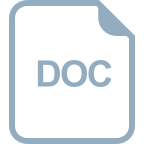
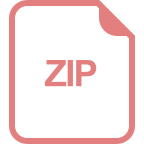
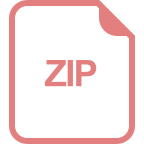
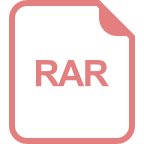
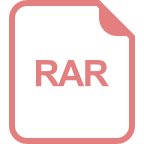
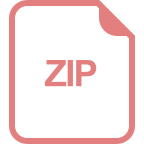
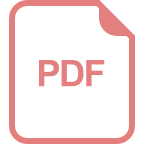
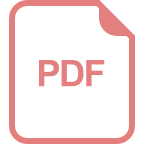
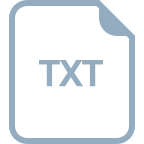
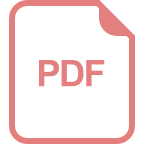
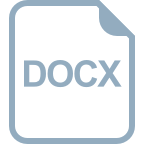
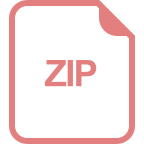