使用elementUI 和 springboot 实现文件下载。文件名与文件地址由后端提供。写完整,不要省略
时间: 2024-01-21 19:18:16 浏览: 76
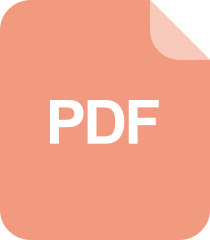
在 Spring Boot 项目中实现文件下载功能

前端代码:
```html
<template>
<div>
<el-button type="primary" @click="handleDownload">下载文件</el-button>
</div>
</template>
<script>
export default {
methods: {
handleDownload() {
const url = 'http://localhost:8080/download'; // 后端提供的文件下载地址
const fileName = 'example.txt'; // 后端提供的文件名
this.$http
.post(url, { fileName }, { responseType: 'blob' }) // 发送POST请求,获取文件流
.then((response) => {
const blob = new Blob([response.data], {
type: response.headers['content-type'],
});
const downloadElement = document.createElement('a');
const href = window.URL.createObjectURL(blob); // 创建下载链接
downloadElement.href = href;
downloadElement.download = fileName;
document.body.appendChild(downloadElement);
downloadElement.click(); // 点击下载链接
document.body.removeChild(downloadElement);
window.URL.revokeObjectURL(href); // 释放资源
});
},
},
};
</script>
```
后端代码:
```java
@RestController
public class FileController {
@PostMapping("/download")
public ResponseEntity<byte[]> download(@RequestBody String fileName) throws IOException {
String filePath = "/path/to/file/" + fileName; // 文件的实际路径
File file = new File(filePath);
byte[] bytes = FileUtils.readFileToByteArray(file);
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", fileName);
return new ResponseEntity<>(bytes, headers, HttpStatus.OK);
}
}
```
需要注意的是,前端使用的是Vue.js框架,通过Vue Resource发送POST请求获取文件流,并通过JavaScript创建下载链接实现文件下载;后端使用的是Spring Boot框架,通过Spring MVC实现文件下载。在后端的代码中,需要注意设置响应头的Content-Disposition属性,以便浏览器能够正确地解析文件名。
阅读全文
相关推荐

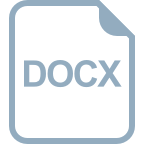
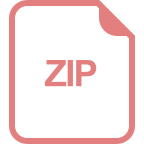
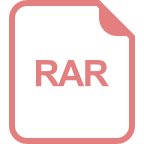
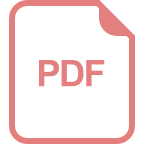
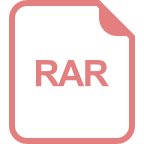
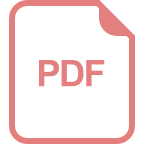
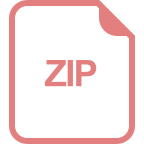
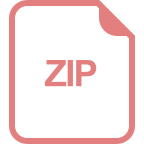
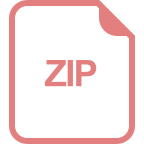
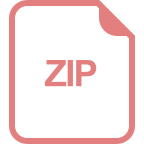
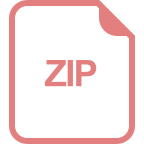
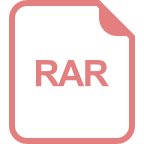
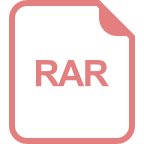
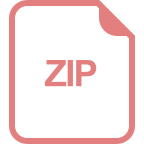
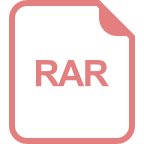