J2ME做无线智能终端,举个例,写段代码
时间: 2024-03-05 10:48:10 浏览: 108
以下是一个简单的 J2ME 代码示例,演示了如何使用 J2ME 编写一个无线智能终端:
```java
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
import javax.microedition.io.*;
import java.io.*;
public class WirelessClient extends MIDlet implements CommandListener {
private static final String SERVER_URL = "socket://192.168.0.1:9999"; // 服务器地址和端口号
private Display display;
private Form mainForm;
private Command exitCommand;
private Command sendCommand;
private TextField messageField;
private StringItem responseItem;
private SocketConnection socketConnection;
private InputStream inputStream;
private OutputStream outputStream;
public void startApp() {
// 创建显示器
display = Display.getDisplay(this);
// 创建主界面
mainForm = new Form("Wireless Client");
// 创建消息输入框和发送按钮
messageField = new TextField("Message", "", 100, TextField.ANY);
sendCommand = new Command("Send", Command.OK, 0);
mainForm.append(messageField);
mainForm.addCommand(sendCommand);
// 创建响应结果显示区域和退出按钮
responseItem = new StringItem("", "");
exitCommand = new Command("Exit", Command.EXIT, 0);
mainForm.append(responseItem);
mainForm.addCommand(exitCommand);
// 显示主界面
display.setCurrent(mainForm);
// 建立与服务器的连接
try {
socketConnection = (SocketConnection) Connector.open(SERVER_URL);
inputStream = socketConnection.openInputStream();
outputStream = socketConnection.openOutputStream();
} catch (IOException e) {
e.printStackTrace();
}
}
public void pauseApp() {}
public void destroyApp(boolean unconditional) {
// 关闭与服务器的连接
try {
inputStream.close();
outputStream.close();
socketConnection.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public void commandAction(Command command, Displayable displayable) {
if (command == exitCommand) {
// 退出应用程序
destroyApp(true);
notifyDestroyed();
} else if (command == sendCommand) {
// 发送消息给服务器
String message = messageField.getString();
try {
outputStream.write(message.getBytes());
outputStream.flush();
// 读取服务器响应
byte[] buffer = new byte[1024];
int len = inputStream.read(buffer);
if (len != -1) {
String response = new String(buffer, 0, len);
responseItem.setText(response);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
这个示例代码实现了一个简单的 J2ME 客户端,该客户端连接到指定的服务器地址和端口号,并可以发送消息到服务器并接收服务器的响应。具体的协议内容处理部分需要根据实际应用情况进行编写。
阅读全文
相关推荐
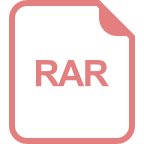
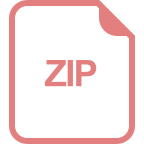
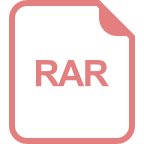
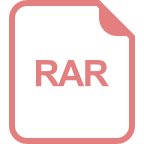
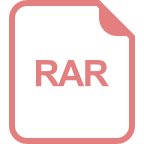
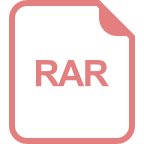
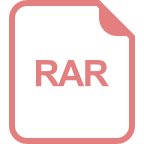
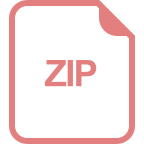
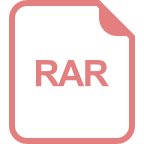
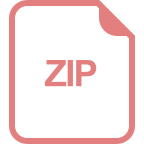
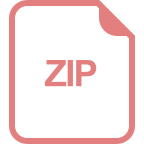
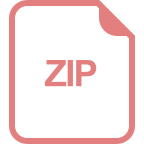
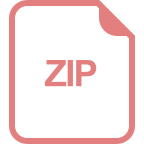
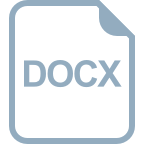