c++中fopen_s报错
时间: 2024-06-12 09:11:19 浏览: 258
在C++中,fopen_s是一个安全的文件操作函数,它可以避免一些潜在的安全问题。如果你在使用fopen_s时出现了报错,可能是因为你的编译器不支持该函数,或者你的代码中存在一些错误。以下是一些可能的解决方案:
1. 将fopen_s替换为fopen。
2. 在项目属性中添加预处理器定义_CRT_SECURE_NO_WARNINGS。
3. 检查你的代码是否存在其他错误,例如文件路径错误或文件名错误。
如果以上方法都无法解决问题,你可以尝试查找更多关于该问题的资料或者咨询相关的技术支持人员。
相关问题
c++ 使用 fopen_s 创建文件
在C++中,`fopen_s`函数是`fopen`的一个安全版本,用于代替旧的、可能存在安全隐患的`fopen`函数,特别是在Windows平台下。当你想要创建一个新的文件时,你可以使用下面的格式:
```cpp
#include <cstdio>
#include <fstream>
int main() {
std::string filename = "example.txt";
char mode[] = "w"; // 或者 "a" (追加模式), "r" (读取模式)等,取决于你需要
std::FILE* file = NULL;
if (_sfsopen_s(&file, filename.c_str(), mode, _TRUNCATE) == 0) { // _sfsopen_s是Windows特有的,_TRUNCATE防止现有内容被保留
if (file != NULL) {
// 文件打开成功,你可以在这里写入数据
std::fprintf(file, "Hello, this is a new file.\n");
fclose(file); // 关闭文件
} else {
// 打开文件失败,处理错误
perror("Error opening file");
}
} else {
// 操作失败,处理安全打开失败的错误
perror("_sfsopen_s error");
}
return 0;
}
```
这里需要注意的是,`_sfsopen_s`和`fopen_s`是Windows API的一部分,而在跨平台环境下可能会有所不同。在非Windows系统上,你可能需要使用标准的`fopen`并配合适当的错误检查。
c++ 用 fopen_s 和fwrite 写入文件
`fopen_s` 和 `fwrite` 是 C++ 中用于文件操作的两个标准库函数,它们分别对应于 C 标准库中的 `fopen` 和 `fwrite` 函数,但在 C++17 及以后的标准中,为了增强对异常处理的支持,引入了安全版本。
`fopen_s` 函数是安全打开文件的函数,它会检查文件是否成功打开,如果发生错误则返回 NULL 或者抛出异常,其原型如下:
```cpp
#include <cstdio>
#include <fstream>
std::FILE* fopen_s(char* filename, size_t n缓冲区大小, const char* mode);
```
要使用它写入文件,你需要先创建一个指向 `FILE` 类型的指针,并尝试打开文件,例如:
```cpp
char filename[] = "example.txt";
std::FILE* file = nullptr;
if (fopen_s(&file, sizeof(filename), "w")) {
std::cerr << "Failed to open file." << std::endl;
// 错误处理...
} else {
// 文件已成功打开
// 使用 fwrite 进行写入
const char data[] = "Hello, this is an example.";
size_t bytes_written = fwrite(data, 1, sizeof(data) - 1, file); // 注意最后一个元素可能有换行符
if (bytes_written != sizeof(data) - 1) {
std::cerr << "Error writing to file." << std::endl;
fclose(file);
} else {
// 写入完成,关闭文件
fclose(file);
}
}
```
`fwrite` 函数用于将数据块写入到指定的位置,它的原型如下:
```cpp
size_t fwrite(const void *ptr, size_t size, size_t count, FILE *stream);
```
阅读全文
相关推荐
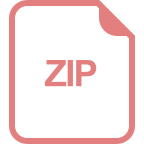
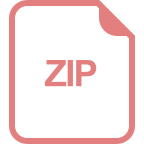













