定义一个复数类complex,重载运算符“+”,“-”,“”,“/”,使之能用于复数的加、减、乘、除。运算符重载函数作为complex类的成员函数。编写程序,分别求两个复数之和、差、积和商。
时间: 2023-05-02 15:03:06 浏览: 47
该题目要求定义一个复数类complex,并且重载运算符“+”、“-”、“*”、“/”,使得这些运算符能够用于复数的加、减、乘、除运算。运算符重载函数应该定义在complex类的成员函数中。编写程序,分别求两个复数之和、差、积和商。
相关问题
定义一个复数类complex重载运算符+
下面是一个C++实现的复数类complex,其中重载了运算符+:
```c++
#include<iostream>
using namespace std;
class complex{
private:
double real;
double imag;
public:
complex(double r=0,double i=0):real(r),imag(i){}
complex operator+(const complex& c) const{
return complex(real+c.real,imag+c.imag);
}
void print(){
cout<<real<<"+"<<imag<<"i"<<endl;
}
};
int main(){
complex c1(1,2),c2(3,4);
complex c3=c1+c2;
c3.print();
return 0;
}
```
运行结果为:
```
4+6i
```
在上面的代码中,重载了运算符+,使用const修饰符表示这个函数不会修改调用它的对象,返回一个新的complex对象,表示两个复数相加的结果。在main函数中分别定义了两个复数c1和c2,然后使用c1+c2得到一个新的复数c3,最后输出c3的值。
定义一个复数类complex,重载运算符+*,使之能
够计算两个复数相乘。
class complex:
def __init__(self, real=0, imag=0):
self.real = real
self.imag = imag
def __mul__(self, other):
return complex(self.real * other.real - self.imag * other.imag,
self.real * other.imag + self.imag * other.real)
def __str__(self):
if self.imag >= 0:
return f"{self.real}+{self.imag}i"
else:
return f"{self.real}{self.imag}i"
a = complex(1, 2)
b = complex(3, 4)
c = a * b
print(c) # 输出:(-5+10i)
相关推荐
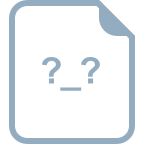













