高响应比优先调度算法C++实现
时间: 2023-10-20 08:09:45 浏览: 120
以下是高响应比优先调度算法C语言实现的示例代码:
```
#include <stdio.h>
#define MAX_PROCESS 10
typedef struct {
int pid;
int arrival_time;
int burst_time;
int waiting_time;
int turnaround_time;
float priority;
int completed;
} Process;
void sort(Process process[], int n) {
// Sort the processes by priority in descending order
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (process[i].priority < process[j].priority) {
Process temp = process[i];
process[i] = process[j];
process[j] = temp;
}
}
}
}
int main() {
int n, total_waiting_time = 0, total_turnaround_time = 0;
printf("Enter the number of processes: ");
scanf("%d", &n);
Process process[MAX_PROCESS];
// Input the process data
for (int i = 0; i < n; i++) {
printf("Enter the arrival time and burst time of process %d: ", i + 1);
scanf("%d%d", &process[i].arrival_time, &process[i].burst_time);
process[i].pid = i + 1;
process[i].completed = 0;
}
// Calculate the priority of each process
for (int i = 0; i < n; i++) {
process[i].priority = 1 + (float)(process[i].waiting_time) / process[i].burst_time;
}
int current_time = 0, completed = 0;
printf("\nGantt Chart:\n");
while (completed != n) {
int highest_priority_index = -1;
float highest_priority = -1;
for (int i = 0; i < n; i++) {
if (process[i].arrival_time <= current_time && !process[i].completed) {
if (process[i].priority > highest_priority) {
highest_priority = process[i].priority;
highest_priority_index = i;
}
}
}
if (highest_priority_index == -1) { // No process available at the moment
printf("idle ");
current_time++;
continue;
}
printf("P%d ", process[highest_priority_index].pid);
process[highest_priority_index].waiting_time = current_time - process[highest_priority_index].arrival_time;
current_time += process[highest_priority_index].burst_time;
process[highest_priority_index].turnaround_time = current_time - process[highest_priority_index].arrival_time;
total_waiting_time += process[highest_priority_index].waiting_time;
total_turnaround_time += process[highest_priority_index].turnaround_time;
process[highest_priority_index].completed = 1;
completed++;
sort(process, n); // Sort the processes again by priority
}
// Print the process table
printf("\n\nProcess Table:\n");
printf("--------------------------------------------------\n");
printf("| Process | Arrival Time | Burst Time | Waiting Time | Turnaround Time |\n");
printf("--------------------------------------------------\n");
for (int i = 0; i < n; i++) {
printf("| P%-6d | %-12d | %-10d | %-12d | %-15d |\n", process[i].pid, process[i].arrival_time, process[i].burst_time, process[i].waiting_time, process[i].turnaround_time);
}
printf("--------------------------------------------------\n");
// Print the average waiting time and turnaround time
printf("\nAverage Waiting Time: %f", (float)total_waiting_time / n);
printf("\nAverage Turnaround Time: %f", (float)total_turnaround_time / n);
return 0;
}
```
该算法的主要思想是根据每个进程的等待时间和执行时间计算优先级,然后按照优先级降序排列进程,选择优先级最高的进程执行,执行完毕后重新计算每个进程的优先级并重新排序,直到所有进程都执行完毕。在实现中,使用了一个结构体来存储每个进程的数据,包括进程ID,到达时间,执行时间,等待时间,周转时间和优先级,以及一个标志位表示进程是否已完成。在计算优先级时,使用了一个公式:优先级 = 1 + 等待时间 / 执行时间。在选择进程执行时,如果有多个进程的优先级相同,则选择到达时间早的进程。最后,根据进程的数据和执行结果,输出进程表和平均等待时间、平均周转时间。
阅读全文
相关推荐
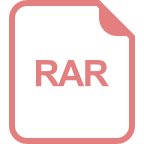
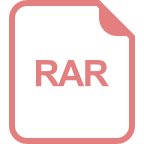

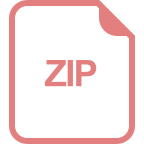
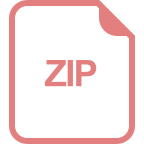
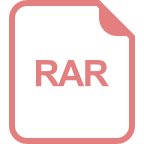







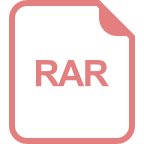
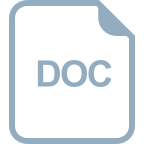
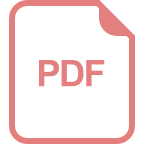
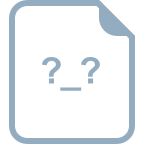