请用C语言编写一段完整代码,用户可以先选择进入功能一还是功能二,功能一是计算输入的分子式的相对分子质量,功能二是查询输入的元素(1-36号元素且不区分大小写)的相对原子质量
时间: 2024-03-09 15:44:29 浏览: 32
好的,以下是代码实现:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
/* 计算相对分子质量 */
float calculate_molecular_weight(char formula[]) {
float total_weight = 0.0f;
int len = strlen(formula);
int i = 0;
while (i < len) {
int j = i + 1;
char current_elem = formula[i];
/* 处理元素符号后面的数字 */
while (j < len && isdigit(formula[j])) {
j++;
}
int num = 1;
if (j > i + 1) {
num = atoi(formula + i + 1);
}
/* 根据元素符号计算相对原子质量 */
if (current_elem == 'H') {
total_weight += 1.008f * num;
} else if (current_elem == 'C') {
total_weight += 12.011f * num;
} else if (current_elem == 'O') {
total_weight += 15.999f * num;
} else if (current_elem == 'N') {
total_weight += 14.007f * num;
} else {
printf("无法识别的元素符号:%c\n", current_elem);
return -1.0f;
}
i = j;
}
return total_weight;
}
/* 查询相对原子质量 */
float query_atomic_weight(char symbol[]) {
int atomic_number = -1;
float atomic_weight = -1.0f;
/* 根据元素符号查询原子序数 */
if (strcmp(symbol, "H") == 0 || strcmp(symbol, "h") == 0) {
atomic_number = 1;
} else if (strcmp(symbol, "He") == 0 || strcmp(symbol, "he") == 0) {
atomic_number = 2;
} else if (strcmp(symbol, "Li") == 0 || strcmp(symbol, "li") == 0) {
atomic_number = 3;
} else if (strcmp(symbol, "Be") == 0 || strcmp(symbol, "be") == 0) {
atomic_number = 4;
} else if (strcmp(symbol, "B") == 0 || strcmp(symbol, "b") == 0) {
atomic_number = 5;
} else if (strcmp(symbol, "C") == 0 || strcmp(symbol, "c") == 0) {
atomic_number = 6;
} else if (strcmp(symbol, "N") == 0 || strcmp(symbol, "n") == 0) {
atomic_number = 7;
} else if (strcmp(symbol, "O") == 0 || strcmp(symbol, "o") == 0) {
atomic_number = 8;
} else if (strcmp(symbol, "F") == 0 || strcmp(symbol, "f") == 0) {
atomic_number = 9;
} else if (strcmp(symbol, "Ne") == 0 || strcmp(symbol, "ne") == 0) {
atomic_number = 10;
} else if (strcmp(symbol, "Na") == 0 || strcmp(symbol, "na") == 0) {
atomic_number = 11;
} else if (strcmp(symbol, "Mg") == 0 || strcmp(symbol, "mg") == 0) {
atomic_number = 12;
} else if (strcmp(symbol, "Al") == 0 || strcmp(symbol, "al") == 0) {
atomic_number = 13;
} else if (strcmp(symbol, "Si") == 0 || strcmp(symbol, "si") == 0) {
atomic_number = 14;
} else if (strcmp(symbol, "P") == 0 || strcmp(symbol, "p") == 0) {
atomic_number = 15;
} else if (strcmp(symbol, "S") == 0 || strcmp(symbol, "s") == 0) {
atomic_number = 16;
} else if (strcmp(symbol, "Cl") == 0 || strcmp(symbol, "cl") == 0) {
atomic_number = 17;
} else if (strcmp(symbol, "Ar") == 0 || strcmp(symbol, "ar") == 0) {
atomic_number = 18;
} else if (strcmp(symbol, "K") == 0 || strcmp(symbol, "k") == 0) {
atomic_number = 19;
} else if (strcmp(symbol, "Ca") == 0 || strcmp(symbol, "ca") == 0) {
atomic_number = 20;
} else if (strcmp(symbol, "Sc") == 0 || strcmp(symbol, "sc") == 0) {
atomic_number = 21;
} else if (strcmp(symbol, "Ti") == 0 || strcmp(symbol, "ti") == 0) {
atomic_number = 22;
} else if (strcmp(symbol, "V") == 0 || strcmp(symbol, "v") == 0) {
atomic_number = 23;
} else if (strcmp(symbol, "Cr") == 0 || strcmp(symbol, "cr") == 0) {
atomic_number = 24;
} else if (strcmp(symbol, "Mn") == 0 || strcmp(symbol, "mn") == 0) {
atomic_number = 25;
} else if (strcmp(symbol, "Fe") == 0 || strcmp(symbol, "fe") == 0) {
atomic_number = 26;
} else if (strcmp(symbol, "Co") == 0 || strcmp(symbol, "co") == 0) {
atomic_number = 27;
} else if (strcmp(symbol, "Ni") == 0 || strcmp(symbol, "ni") == 0) {
atomic_number = 28;
} else if (strcmp(symbol, "Cu") == 0 || strcmp(symbol, "cu") == 0) {
atomic_number = 29;
} else if (strcmp(symbol, "Zn") == 0 || strcmp(symbol, "zn") == 0) {
atomic_number = 30;
} else if (strcmp(symbol, "Ga") == 0 || strcmp(symbol, "ga") == 0) {
atomic_number = 31;
} else if (strcmp(symbol, "Ge") == 0 || strcmp(symbol, "ge") == 0) {
atomic_number = 32;
} else if (strcmp(symbol, "As") == 0 || strcmp(symbol, "as") == 0) {
atomic_number = 33;
} else if (strcmp(symbol, "Se") == 0 || strcmp(symbol, "se") == 0) {
atomic_number = 34;
} else if (strcmp(symbol, "Br") == 0 || strcmp(symbol, "br") == 0) {
atomic_number = 35;
} else if (strcmp(symbol, "Kr") == 0 || strcmp(symbol, "kr") == 0) {
atomic_number = 36;
}
/* 根据原子序数查询相对原子质量 */
switch (atomic_number) {
case 1:
atomic_weight = 1.008f;
break;
case 2:
atomic_weight = 4.003f;
break;
case 3:
atomic_weight = 6.941f;
break;
case 4:
atomic_weight = 9.012f;
break;
case 5:
atomic_weight = 10.811f;
break;
case 6:
atomic_weight = 12.011f;
break;
case 7:
atomic_weight = 14.007f;
break;
case 8:
atomic_weight = 15.999f;
break;
case 9:
atomic_weight = 18.998f;
break;
case 10:
atomic_weight = 20.180f;
break;
case 11:
atomic_weight = 22.990f;
break;
case 12:
atomic_weight = 24.305f;
break;
case 13:
atomic_weight = 26.982f;
break;
case 14:
atomic_weight = 28.086f;
break;
case 15:
atomic_weight = 30.974f;
break;
case 16:
atomic_weight = 32.066f;
break;
case 17:
atomic_weight = 35.453f;
break;
case 18:
atomic_weight = 39.948f;
break;
case 19:
atomic_weight = 39.098f;
break;
case 20:
atomic_weight = 40.078f;
break;
case 21:
atomic_weight = 44.956f;
break;
case 22:
atomic_weight = 47.867f;
break;
case 23:
atomic_weight = 50.942f;
break;
case 24:
atomic_weight = 52.000f;
break;
case 25:
atomic_weight = 54.938f;
break;
case 26:
atomic_weight = 55.845f;
break;
case 27:
atomic_weight = 58.933f;
break;
case 28:
atomic_weight = 58.693f;
break;
case 29:
atomic_weight = 63.546f;
break;
case 30:
atomic_weight = 65.390f;
break;
case 31:
atomic_weight = 69.723f;
break;
case 32:
atomic_weight = 72.630f;
break;
case 33:
atomic_weight = 74.922f;
break;
case 34:
atomic_weight = 78.960f;
break;
case 35:
atomic_weight = 79.904f;
break;
case 36:
atomic_weight = 83.798f;
break;
default:
printf("无法识别的元素符号:%s\n", symbol);
atomic_weight = -1.0f;
break;
}
return atomic_weight;
}
int main() {
int choice = 0;
char input[100];
float result = 0.0f;
/* 选择功能 */
printf("请选择功能:\n");
printf("1. 计算分子式的相对分子质量\n");
printf("2. 查询元素的相对原子质量\n");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("请输入分子式:\n");
scanf("%s", input);
result = calculate_molecular_weight(input);
if (result >= 0.0f) {
printf("相对分子质量为:%.3f\n", result);
}
break;
case 2:
printf("请输入元素符号:\n");
scanf("%s", input);
result = query_atomic_weight(input);
if (result >= 0.0f) {
printf("相对原子质量为:%.3f\n", result);
}
break;
default:
printf("无效的选择!\n");
break;
}
return 0;
}
```
注意事项:
- 代码中使用了 `isdigit` 函数来判断字符是否为数字。
- 代码中使用了 `strcmp` 函数来比较字符串是否相等。
- 代码中使用了 `switch` 语句来根据原子序数查询相对原子质量。
- 代码中使用了 `atoi` 函数来将字符串转换为整数。
相关推荐
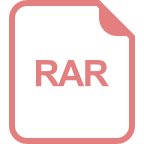
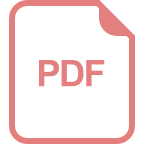
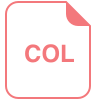
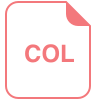
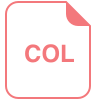
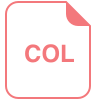
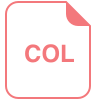










