C++ 识别有效的IP地址和掩码并进行分类统计
时间: 2024-05-13 22:17:48 浏览: 17
下面是一个简单的 C++ 代码示例,可以识别有效的IP地址和掩码,并对它们进行分类统计。该程序将从标准输入中读取IP地址和掩码,然后将它们分类为A、B、C、D或无效,并输出每个类别的计数。
```cpp
#include <iostream>
#include <string>
#include <regex>
using namespace std;
int main() {
int countA = 0, countB = 0, countC = 0, countD = 0, countInvalid = 0;
regex ipPattern("^\\d{1,3}\\.\\d{1,3}\\.\\d{1,3}\\.\\d{1,3}$");
regex maskPattern("^\\d{1,2}$");
while (true) {
string ip, mask;
cin >> ip >> mask;
// Exit loop when input is empty
if (ip.empty() || mask.empty()) {
break;
}
// Check if IP address and mask are valid
if (!regex_match(ip, ipPattern) || !regex_match(mask, maskPattern)) {
countInvalid++;
continue;
}
// Split IP address into octets
int octets[4];
sscanf(ip.c_str(), "%d.%d.%d.%d", &octets[0], &octets[1], &octets[2], &octets[3]);
// Classify IP address based on first octet
if (octets[0] >= 1 && octets[0] <= 126) {
countA++;
} else if (octets[0] >= 128 && octets[0] <= 191) {
countB++;
} else if (octets[0] >= 192 && octets[0] <= 223) {
countC++;
} else if (octets[0] >= 224 && octets[0] <= 239) {
countD++;
} else {
countInvalid++;
}
}
// Output results
cout << "Class A: " << countA << endl;
cout << "Class B: " << countB << endl;
cout << "Class C: " << countC << endl;
cout << "Class D: " << countD << endl;
cout << "Invalid: " << countInvalid << endl;
return 0;
}
```
该程序使用正则表达式验证了IP地址和掩码的格式,并使用 sscanf() 函数将IP地址拆分为其四个八位组。然后,它根据第一个八位组将IP地址分类为A、B、C、D或无效,并计算每个类别的计数。最后,程序输出每个类别的计数。
相关推荐
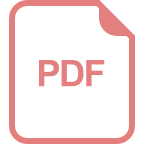














