用C语言给我写一个堆排序算法
时间: 2023-05-27 22:07:09 浏览: 69
#include <stdio.h>
#include <stdlib.h>
// 交换两个数的值
void swap(int *a, int *b)
{
int temp = *a;
*a = *b;
*b = temp;
}
// 调整堆
void adjust_heap(int *arr, int parent, int len)
{
int child = parent * 2 + 1; // 左孩子节点
int temp = arr[parent]; // 父节点的值
while (child < len)
{
if (child + 1 < len && arr[child] < arr[child + 1])
{
child++; // 取左右孩子节点的最大值
}
if (temp >= arr[child])
{
break;
}
arr[parent] = arr[child];
parent = child;
child = parent * 2 + 1;
}
arr[parent] = temp;
}
// 堆排序
void heap_sort(int *arr, int len)
{
// 构建大根堆
for (int i = len / 2 - 1; i >= 0; i--)
{
adjust_heap(arr, i, len);
}
// 排序
for (int i = len - 1; i > 0; i--)
{
swap(&arr[0], &arr[i]); // 将当前最大值放到数组末尾
adjust_heap(arr, 0, i); // 调整剩余元素
}
}
int main()
{
int arr[] = { 6, 5, 3, 1, 8, 7, 2, 4 };
int len = sizeof(arr) / sizeof(arr[0]);
heap_sort(arr, len);
for (int i = 0; i < len; i++)
{
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
相关推荐
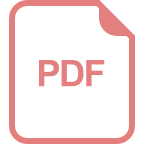














