C语言三个线程循环打印ABC
时间: 2023-06-22 09:48:41 浏览: 76
在C语言中,可以使用`pthread`库来实现多线程。具体代码如下:
```c
#include <stdio.h>
#include <pthread.h>
pthread_mutex_t a_lock, b_lock, c_lock;
void* print_thread(void* arg) {
int id = *(int*)arg;
int i;
for (i = 0; i < 5; i++) {
if (id == 0) {
pthread_mutex_lock(&c_lock);
printf("A");
pthread_mutex_unlock(&a_lock);
} else if (id == 1) {
pthread_mutex_lock(&a_lock);
printf("B");
pthread_mutex_unlock(&b_lock);
} else {
pthread_mutex_lock(&b_lock);
printf("C");
pthread_mutex_unlock(&c_lock);
}
}
pthread_exit(NULL);
}
int main() {
pthread_t threads[3];
int ids[3] = {0, 1, 2};
pthread_mutex_init(&a_lock, NULL);
pthread_mutex_init(&b_lock, NULL);
pthread_mutex_init(&c_lock, NULL);
pthread_mutex_lock(&a_lock);
pthread_mutex_lock(&b_lock);
pthread_create(&threads[0], NULL, print_thread, &ids[0]);
pthread_create(&threads[1], NULL, print_thread, &ids[1]);
pthread_create(&threads[2], NULL, print_thread, &ids[2]);
pthread_join(threads[0], NULL);
pthread_join(threads[1], NULL);
pthread_join(threads[2], NULL);
pthread_mutex_destroy(&a_lock);
pthread_mutex_destroy(&b_lock);
pthread_mutex_destroy(&c_lock);
return 0;
}
```
其中,定义了一个`print_thread`函数,用于执行打印操作。每个线程需要持有前一个线程的锁和自己的锁,当前一个线程执行完后,释放前一个锁,再获取自己的锁,进行打印操作。最后,主线程等待所有线程执行完毕后,销毁锁并退出程序。
相关推荐
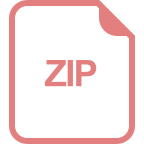
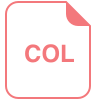
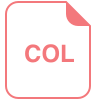
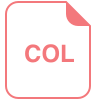
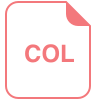
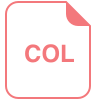









