实现一个和Winform一样的NumericUpDown控件
时间: 2024-03-05 16:50:24 浏览: 40
可以使用 WPF 中的自定义控件来实现类似 WinForm 中的 NumericUpDown 控件。下面是一个简单的实现:
首先,创建一个新的 UserControl,命名为 NumericUpDown。在 UserControl 中添加两个 Button 控件,一个 TextBox 控件和一个 Label 控件,如下所示:
```xml
<UserControl x:Class="WpfApp1.NumericUpDown"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
d:DesignHeight="30" d:DesignWidth="120">
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*" />
<ColumnDefinition Width="Auto" />
</Grid.ColumnDefinitions>
<TextBox x:Name="TextBox" Grid.Column="0" />
<Grid Grid.Column="1">
<Grid.RowDefinitions>
<RowDefinition Height="*" />
<RowDefinition Height="*" />
</Grid.RowDefinitions>
<Button x:Name="UpButton" Grid.Row="0" Content="▲" Click="UpButton_Click" />
<Button x:Name="DownButton" Grid.Row="1" Content="▼" Click="DownButton_Click" />
</Grid>
<Label x:Name="Label" Content="Label" HorizontalAlignment="Left" VerticalAlignment="Top" Margin="0,-20,0,0" />
</Grid>
</UserControl>
```
然后,在 NumericUpDown.xaml.cs 文件中添加以下代码:
```csharp
public partial class NumericUpDown : UserControl
{
public NumericUpDown()
{
InitializeComponent();
}
public static readonly DependencyProperty ValueProperty = DependencyProperty.Register("Value", typeof(double), typeof(NumericUpDown), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.BindsTwoWayByDefault, ValueChangedCallback));
public static readonly DependencyProperty MinimumProperty = DependencyProperty.Register("Minimum", typeof(double), typeof(NumericUpDown), new PropertyMetadata(double.MinValue));
public static readonly DependencyProperty MaximumProperty = DependencyProperty.Register("Maximum", typeof(double), typeof(NumericUpDown), new PropertyMetadata(double.MaxValue));
public double Value
{
get { return (double)GetValue(ValueProperty); }
set { SetValue(ValueProperty, value); }
}
public double Minimum
{
get { return (double)GetValue(MinimumProperty); }
set { SetValue(MinimumProperty, value); }
}
public double Maximum
{
get { return (double)GetValue(MaximumProperty); }
set { SetValue(MaximumProperty, value); }
}
private static void ValueChangedCallback(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
var numericUpDown = d as NumericUpDown;
if (numericUpDown != null)
{
numericUpDown.TextBox.Text = e.NewValue.ToString();
}
}
private void UpButton_Click(object sender, RoutedEventArgs e)
{
var newValue = Value + 1.0;
if (newValue <= Maximum)
{
Value = newValue;
}
}
private void DownButton_Click(object sender, RoutedEventArgs e)
{
var newValue = Value - 1.0;
if (newValue >= Minimum)
{
Value = newValue;
}
}
}
```
这里我们创建了 Value、Minimum 和 Maximum 三个依赖属性,分别表示当前值、最小值和最大值。当 Value 属性改变时,我们将文本框中的值更新为新值。当 UpButton 或 DownButton 被单击时,我们检查新值是否在最小值和最大值之间。如果是,则更新 Value 属性。最后,可以在 MainWindow.xaml 中使用 NumericUpDown 控件:
```xml
<StackPanel>
<Label>Value:</Label>
<local:NumericUpDown Value="0" Minimum="-10" Maximum="10" />
</StackPanel>
```
相关推荐
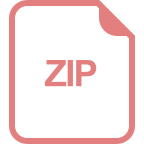














