c语言实现二叉树链前序遍历搜查数据的代码
时间: 2023-12-04 12:02:30 浏览: 22
可以参考以下代码,实现C语言中二叉树链前序遍历搜查数据的功能:
```
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
void preOrderTraversal(struct TreeNode* root) {
if (root == NULL) {
return;
}
printf("%d ", root->val);
preOrderTraversal(root->left);
preOrderTraversal(root->right);
}
struct TreeNode* searchBST(struct TreeNode* root, int val){
if (root == NULL || root->val == val) {
return root;
}
if (val < root->val) {
return searchBST(root->left, val);
} else {
return searchBST(root->right, val);
}
}
int main() {
struct TreeNode* root = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->val = 4;
root->left = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->left->val = 2;
root->left->left = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->left->left->val = 1;
root->left->right = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->left->right->val = 3;
root->right = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->right->val = 7;
root->right->left = NULL;
root->right->right = NULL;
printf("Preorder traversal of binary tree: ");
preOrderTraversal(root);
int target = 3;
struct TreeNode* res = searchBST(root, target);
if (res == NULL) {
printf("\n%d not found in the BST.\n", target);
} else {
printf("\n%d found in the BST.\n", res->val);
}
return 0;
}
```
希望这段代码能满足你的需求!有其他问题欢迎继续提问。
相关推荐
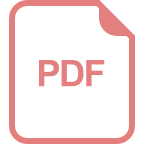
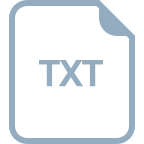
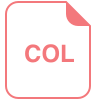
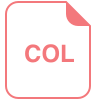
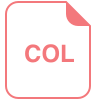
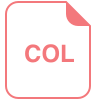
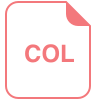









