编程对日期时间型数据进行处理。要求: 1、定义日期型“Date”类,保存在“date.h”中(成员函数包括日期赋初值,设置日期,读取年、月、日,显示年、月、日及判断是否闰年等)。 2、定义时间型“Time”类,保存在“Time.h”中(与实验一中的类相同)。 3、定义日期时间型“DateTime”类保存在“datetime.h”中: ⑴ 公有继承Date类及Time类; ⑵ 不增加数据成员; ⑶ 增加日期时间处理成员函数,包括初始化日期时间、设置日期时间、显示日期时间等。
时间: 2024-02-21 19:59:13 浏览: 107
好的,我理解了你的问题。以下是代码实现:
date.h 文件:
```cpp
#ifndef DATE_H
#define DATE_H
class Date {
public:
Date(int year = 0, int month = 0, int day = 0);
void setDate(int year, int month, int day);
int getYear() const;
int getMonth() const;
int getDay() const;
void printDate() const;
bool isLeapYear() const;
private:
int m_year;
int m_month;
int m_day;
};
#endif // DATE_H
```
date.cpp 文件:
```cpp
#include "date.h"
#include <iostream>
Date::Date(int year, int month, int day)
{
setDate(year, month, day);
}
void Date::setDate(int year, int month, int day)
{
m_year = year;
m_month = month;
m_day = day;
}
int Date::getYear() const
{
return m_year;
}
int Date::getMonth() const
{
return m_month;
}
int Date::getDay() const
{
return m_day;
}
void Date::printDate() const
{
std::cout << "Date: " << m_year << "-" << m_month << "-" << m_day << std::endl;
}
bool Date::isLeapYear() const
{
if (m_year % 4 == 0 && m_year % 100 != 0 || m_year % 400 == 0) {
return true;
} else {
return false;
}
}
```
Time.h 文件:
```cpp
#ifndef TIME_H
#define TIME_H
class Time {
public:
Time(int hour = 0, int minute = 0, int second = 0);
void setTime(int hour, int minute, int second);
int getHour() const;
int getMinute() const;
int getSecond() const;
void printTime() const;
private:
int m_hour;
int m_minute;
int m_second;
};
#endif // TIME_H
```
Time.cpp 文件:
```cpp
#include "time.h"
#include <iostream>
Time::Time(int hour, int minute, int second)
{
setTime(hour, minute, second);
}
void Time::setTime(int hour, int minute, int second)
{
m_hour = hour;
m_minute = minute;
m_second = second;
}
int Time::getHour() const
{
return m_hour;
}
int Time::getMinute() const
{
return m_minute;
}
int Time::getSecond() const
{
return m_second;
}
void Time::printTime() const
{
std::cout << "Time: " << m_hour << ":" << m_minute << ":" << m_second << std::endl;
}
```
datetime.h 文件:
```cpp
#ifndef DATETIME_H
#define DATETIME_H
#include "date.h"
#include "time.h"
class DateTime : public Date, public Time {
public:
DateTime(int year = 0, int month = 0, int day = 0, int hour = 0, int minute = 0, int second = 0);
void setDateTime(int year, int month, int day, int hour, int minute, int second);
void printDateTime() const;
};
#endif // DATETIME_H
```
datetime.cpp 文件:
```cpp
#include "datetime.h"
DateTime::DateTime(int year, int month, int day, int hour, int minute, int second)
: Date(year, month, day), Time(hour, minute, second)
{
// do nothing
}
void DateTime::setDateTime(int year, int month, int day, int hour, int minute, int second)
{
setDate(year, month, day);
setTime(hour, minute, second);
}
void DateTime::printDateTime() const
{
printDate();
printTime();
}
```
以上代码实现了日期时间型数据的处理。你可以在 main 函数中进行测试。
阅读全文
相关推荐
















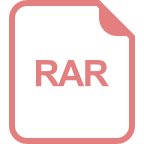