axios 跨域配置
时间: 2023-06-29 10:19:41 浏览: 138
在使用 axios 进行跨域请求时,需要进行一些配置,以下是一个基本的跨域配置示例:
```javascript
import axios from 'axios'
axios.defaults.baseURL = 'http://api.example.com'
axios.defaults.withCredentials = true
axios.defaults.headers.common['Authorization'] = 'Bearer ' + localStorage.getItem('token')
axios.interceptors.response.use(
response => {
return response
},
error => {
if (error.response.status === 401) {
// 处理未授权的情况
console.log('Unauthorized')
}
return Promise.reject(error)
}
)
```
其中,`baseURL` 是你要请求的域名,`withCredentials` 表示是否允许发送跨域 cookie,`headers` 中可以设置公共的请求头,例如上面的示例中设置了一个 Bearer Token。另外,`interceptors` 中可以对响应进行拦截处理,例如处理未授权的情况。
相关问题
axios跨域代理配置
可以尝试在配置文件中添加以下代码:
```javascript
module.exports = {
devServer: {
// ...
proxy: {
'/api': {
target: 'http://api.example.com', // 实际要请求的服务器URL
changeOrigin: true, // 允许跨域
pathRewrite: { // 重写路径
'^/api': ''
}
}
}
}
}
```
这样,在前端请求后端API时,只需要将请求的路径改为`/api`开头即可。比如请求`http://api.example.com/test`,可以写成`/api/test`,axios会将`/api`重写为实际请求的服务器URL,并加上`/test`,从而实现跨域请求。
vue2配置axios跨域
在Vue2中配置axios跨域可以通过以下步骤实现:
1. 安装axios:首先,在你的Vue项目中安装axios。可以使用npm或者yarn命令进行安装,例如:
```
npm install axios
```
2. 创建代理配置:在Vue项目的根目录下,创建一个vue.config.js文件(如果已经存在则跳过此步骤)。在该文件中添加以下代码:
```javascript
module.exports = {
devServer: {
proxy: {
'/api': {
target: 'http://api.example.com', // 设置你想要请求的后端接口地址
changeOrigin: true,
pathRewrite: {
'^/api': '' // 如果你的后端接口没有/api前缀,可以将此行注释掉
}
}
}
}
}
```
这里的配置表示将以`/api`开头的请求代理到`http://api.example.com`,你可以根据实际情况修改目标地址。
3. 使用axios发送请求:在你的Vue组件中,可以使用axios发送跨域请求。例如:
```javascript
import axios from 'axios'
axios.get('/api/data')
.then(response => {
console.log(response.data)
})
.catch(error => {
console.error(error)
})
```
这里的`/api/data`是相对于你的前端服务器的路径,实际上会被代理到后端接口地址。
相关推荐
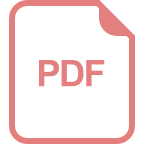
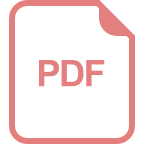
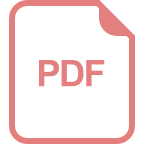
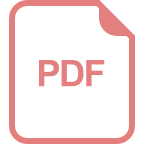
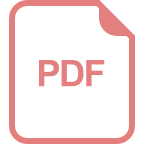
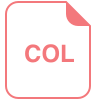
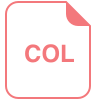
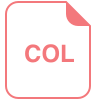
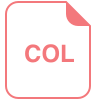
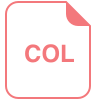





