帮我选择合适的数据结构并用c++语言写一个程序,要求是设计并实现一个学生信息管理系统(学生人数不超过100),即定义一个包含学生信息(学号,姓名,成绩)的顺序表,可以不考虑重名的情况,系统至少包含以下功能: (1) 根据指定学生个数,逐个输入学生信息; (2) 逐个显示学生表中所有学生的相关信息; (3) 给定一个学生信息,插入到表中指定的位置; (4) 删除指定位置的学生记录; (5) 统计表中学生个数; (6) 按照姓名(直接插入排序)、学号(快速排序)进行排序; (7) 根据学号(折半查找)进行查找,如果成功,返回此学生的姓名和成绩。
时间: 2023-12-24 14:04:27 浏览: 210
以下是一个基于顺序表的学生信息管理系统的C++实现代码:
```cpp
#include <iostream>
#include <algorithm>
#include <string>
using namespace std;
// 定义学生结构体
struct Student {
string id; // 学号
string name; // 姓名
int score; // 成绩
};
// 定义学生信息管理类
class StudentManager {
public:
StudentManager() : size(0) {}
void insert(Student s, int pos) {
if (size == MAX_SIZE) {
cout << "Error: the student table is full." << endl;
return;
}
if (pos < 1 || pos > size + 1) {
cout << "Error: the position is invalid." << endl;
return;
}
for (int i = size; i >= pos; i--) {
students[i] = students[i - 1];
}
students[pos - 1] = s;
size++;
}
void remove(int pos) {
if (pos < 1 || pos > size) {
cout << "Error: the position is invalid." << endl;
return;
}
for (int i = pos - 1; i < size - 1; i++) {
students[i] = students[i + 1];
}
size--;
}
void display() {
cout << "Student table:" << endl;
for (int i = 0; i < size; i++) {
cout << students[i].id << " " << students[i].name << " " << students[i].score << endl;
}
}
void sortById() {
sort(students, students + size, [](Student a, Student b) {
return a.id < b.id;
});
}
void sortByName() {
sort(students, students + size, [](Student a, Student b) {
return a.name < b.name;
});
}
int searchById(string id) {
int left = 0, right = size - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (students[mid].id == id) {
return mid;
} else if (students[mid].id < id) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
int count() {
return size;
}
private:
static const int MAX_SIZE = 100;
Student students[MAX_SIZE];
int size;
};
int main() {
StudentManager manager;
// 插入学生信息
cout << "Please input the number of students: ";
int n;
cin >> n;
for (int i = 0; i < n; i++) {
Student s;
cout << "Please input the information of student " << i + 1 << ": ";
cin >> s.id >> s.name >> s.score;
manager.insert(s, i + 1);
}
// 显示学生信息
manager.display();
// 删除学生信息
cout << "Please input the position of the student you want to remove: ";
int pos;
cin >> pos;
manager.remove(pos);
manager.display();
// 统计学生个数
cout << "The number of students is: " << manager.count() << endl;
// 按照姓名排序
manager.sortByName();
manager.display();
// 按照学号排序
manager.sortById();
manager.display();
// 根据学号查找学生信息
cout << "Please input the id of the student you want to search: ";
string id;
cin >> id;
int index = manager.searchById(id);
if (index != -1) {
cout << "The information of the student is: " << manager[index].name << " " << manager[index].score << endl;
} else {
cout << "The student is not found." << endl;
}
return 0;
}
```
该程序使用一个包含100个元素的顺序表来存储学生信息,主要包括以下功能:
1. 插入学生信息
2. 删除学生信息
3. 显示学生信息
4. 统计学生个数
5. 按照姓名排序
6. 按照学号排序
7. 根据学号查找学生信息
其中,插入、删除、统计学生个数、按照姓名排序和按照学号排序均使用顺序表的基本操作来实现;查找学生信息使用折半查找算法来实现。
阅读全文
相关推荐
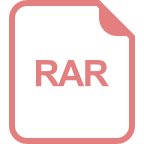
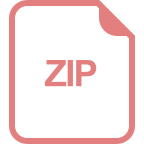
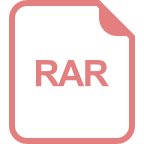
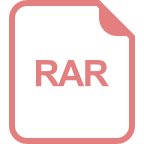
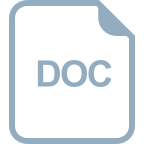
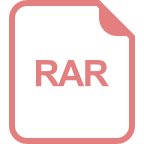
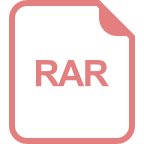
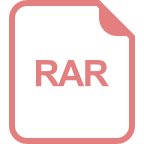
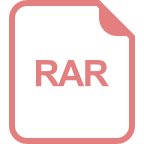
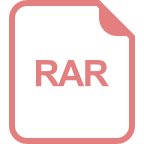
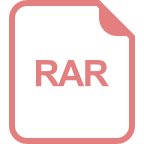
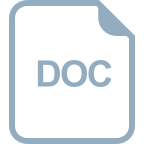
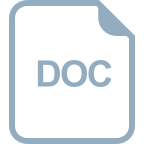
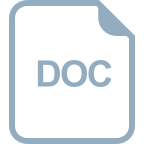
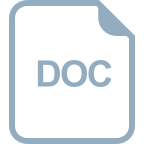