基于链式存储结构的图书表的创建,新书入库listinsert,旧书出库,定义图书表的输出函数outputlist最爱图书查找listlove,编写main函数
时间: 2024-10-16 16:10:47 浏览: 32
好的,我们可以按照以下步骤来设计这个基于链表的图书管理系统:
首先,我们需要定义一个`Book`结构体,用于表示图书,包含书名、作者、库存量等信息。然后,我们使用链表作为存储结构。
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Book {
char *title;
char *author;
int stock;
struct Book* next;
} Book;
// 创建一个新的图书节点
Book* createBook(char* title, char* author, int stock) {
Book* new_book = (Book*)malloc(sizeof(Book));
if (!new_book) {
printf("Memory allocation failed.\n");
return NULL;
}
new_book->title = strdup(title);
new_book->author = strdup(author);
new_book->stock = stock;
new_book->next = NULL;
return new_book;
}
// 新书入库函数(插入到链表尾部)
void listInsert(Book** head, char* title, char* author, int stock) {
Book* new_book = createBook(title, author, stock);
if (!new_book) return;
if (*head == NULL) {
*head = new_book;
return;
}
Book* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = new_book;
}
// 旧书出库
void bookOut库(Book** head, const char* title) {
Book* current = *head;
Book* previous = NULL;
while (current != NULL) {
if (strcmp(current->title, title) == 0) {
if (previous == NULL) {
*head = current->next;
} else {
previous->next = current->next;
}
free(current->title);
free(current->author);
free(current);
return;
}
previous = current;
current = current->next;
}
printf("Book not found.\n");
}
// 定义图书表的输出函数
void outputList(Book* head) {
if (head == NULL) {
printf("Empty book list.\n");
return;
}
printf("Title\tAuthor\tStock\n");
Book* current = head;
while (current != NULL) {
printf("%s\t%s\t%d\n", current->title, current->author, current->stock);
current = current->next;
}
}
// 查找最爱图书函数(这里假设有一个全局变量存储最爱图书)
char* findFavorite(Book* head) {
// 假设favorite_title存在并已经初始化
// 实际操作根据数据结构调整,例如使用哈希表或优先级队列
return favorite_title;
}
int main() {
// 初始化链表为空
Book* head = NULL;
// 插入书籍
listInsert(&head, "Book1", "Author1", 50);
listInsert(&head, "Book2", "Author2", 30);
// 输出列表
outputList(head);
// 模拟出库(例如最爱图书被借走)
char* loved_title = findFavorite(head); // 假设loved_title为"Book1"
bookOut库(&head, loved_title);
// 再次输出列表检查是否正确减少库存
outputList(head);
// 清理内存
while (head != NULL) {
Book* temp = head;
head = head->next;
free(temp);
}
return 0;
}
```
阅读全文
相关推荐
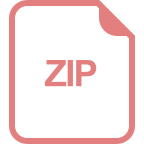
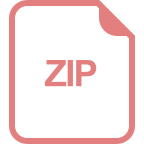
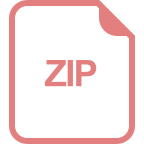






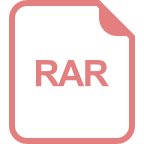
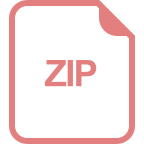
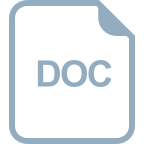


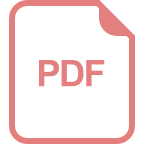
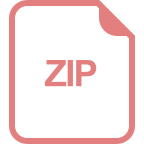
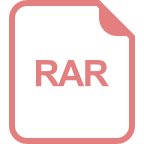
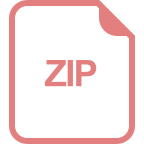