java8发送post请求,返回Json包体,格式为 application/json 并带有请求头
时间: 2024-09-10 10:08:53 浏览: 44
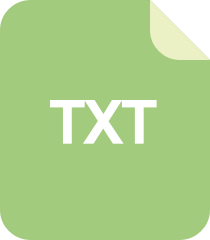
java发送http请求报文json
在Java 8中,你可以使用多种方法来发送一个带有JSON格式体的POST请求,同时携带特定的请求头。以下是一个使用Java标准库中的`java.net.HttpURLConnection`类的示例:
```java
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.charset.StandardCharsets;
public class PostRequestExample {
public static void sendPostRequest(String targetUrl, String jsonPayload) throws Exception {
// 创建URL对象
URL url = new URL(targetUrl);
// 打开连接
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
// 设置请求方法为POST
conn.setRequestMethod("POST");
// 设置请求头,告诉服务器发送的内容类型为JSON
conn.setRequestProperty("Content-Type", "application/json; utf-8");
// 设置请求头,告诉服务器接受的内容类型为JSON
conn.setRequestProperty("Accept", "application/json");
// 允许输出
conn.setDoOutput(true);
// 发送请求
try (OutputStream os = conn.getOutputStream()) {
// 将JSON数据写入输出流
byte[] input = jsonPayload.getBytes(StandardCharsets.UTF_8);
os.write(input, 0, input.length);
}
// 获取响应码
int responseCode = conn.getResponseCode();
System.out.println("POST Response Code :: " + responseCode);
// 处理响应
if (responseCode == HttpURLConnection.HTTP_OK) { //success
// 读取响应
String response = new BufferedReader(new InputStreamReader(conn.getInputStream()))
.lines().collect(Collectors.joining(System.lineSeparator()));
System.out.println("Response from server: " + response);
} else {
System.out.println("POST request not worked");
}
// 断开连接
conn.disconnect();
}
public static void main(String[] args) {
String targetUrl = "http://your-endpoint.com/api/endpoint";
String jsonPayload = "{\"key1\":\"value1\",\"key2\":\"value2\"}";
try {
sendPostRequest(targetUrl, jsonPayload);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在这个例子中,我们首先创建了一个`HttpURLConnection`对象,然后设置了请求方法为POST,并设置了请求头。我们通过`setDoOutput(true)`来指示我们将会向服务器发送数据。然后,我们使用`getOutputStream()`来写入JSON格式的请求体。最后,我们读取服务器的响应。
请注意,你需要替换`targetUrl`为你的实际API端点,并根据需要修改`jsonPayload`变量的内容。
阅读全文
相关推荐
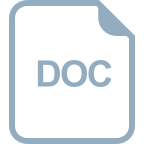
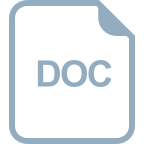
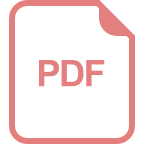
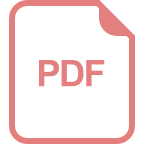
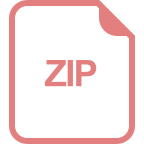
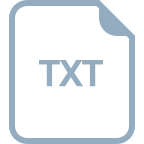
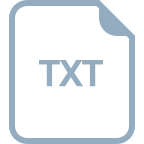
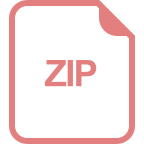
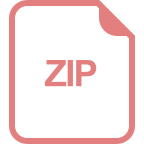
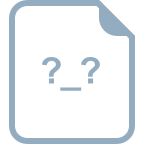
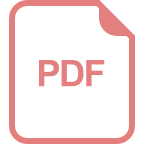
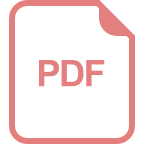
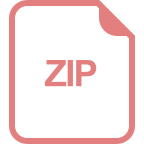
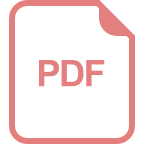
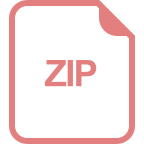
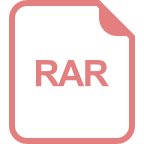
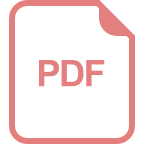
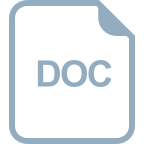